Python Data Structures and Algorithms - Recursion: Convert an integer to a string in any base
2. Integer to String Conversion in Any Base Using Recursion
Write a Python program to convert an integer to a string in any base using recursion.
Sample Solution:
Python Code:
# Define a function named to_string that converts a number 'n' to a string representation
# in a given 'base' using a character set "0123456789ABCDEF"
def to_string(n, base):
# Define a character set for the conversion in hexadecimal format
conver_tString = "0123456789ABCDEF"
# Check if the number 'n' is less than the specified base
if n < base:
# If 'n' is less than the base, return the corresponding character from the character set
return conver_tString[n]
else:
# If 'n' is greater than or equal to the base, recursively call the to_string function
# to convert the quotient (n // base) to a string and concatenate it with the remainder
# (n % base) represented in the character set
return to_string(n // base, base) + conver_tString[n % base]
# Print the result of calling the to_string function with the input values 2835 and 16
print(to_string(2835, 16))
Sample Output:
B13
Flowchart:
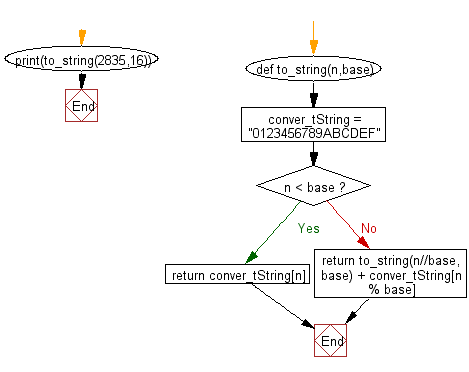
For more Practice: Solve these Related Problems:
- Write a Python program to convert a positive integer to a string in a given base (2–36) recursively.
- Write a Python program to implement a recursive function that converts negative integers to their string representation in any base.
- Write a Python program to convert an integer to its base-n representation using tail recursion.
- Write a Python program to recursively convert an integer to a string in any base and output the result in uppercase for bases above 10.
Go to:
Previous: Write a Python program to calculate the sum of a list of numbers.
Next: Write a Python program of recursion list sum.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.