Python: Convert a string into datetime
49. String to Datetime Conversion
Write a Python program to convert a string into datetime.
Sample Solution:
Python Code:
# Import the datetime class from the datetime module
from datetime import datetime
# Parse the string 'May 12 2016 2:25AM' into a datetime object using the specified format
date_obj = datetime.strptime('May 12 2016 2:25AM', '%b %d %Y %I:%M%p')
# Print an empty line for formatting purposes
print()
# Print the datetime object
print(date_obj)
# Print an empty line for formatting purposes
print()
Output:
2016-05-12 02:25:00
Explanation:
In the exercise above,
- The code imports the "datetime" class from the "datetime" module.
- It uses the "strptime()" method of the "datetime" class to parse the string 'May 12 2016 2:25AM' into a datetime object.
- The first argument is the string to be parsed.
- The second argument is the format string specifying the format of the input string.
- It prints an empty line for formatting purposes.
- It prints the datetime object.
- It prints an empty line for formatting purposes.
Flowchart:
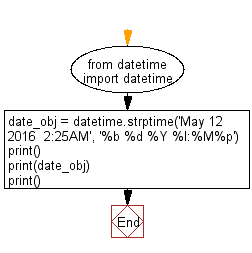
For more Practice: Solve these Related Problems:
- Write a Python program to convert multiple date strings in different formats to datetime objects and then sort them chronologically.
- Write a Python script to parse a string containing a date and time with a timezone and convert it to a naive datetime object.
- Write a Python function to convert a date string with abbreviated month names to a datetime object and then output it in "YYYY-MM-DD HH:MM" format.
- Write a Python program to transform a list of date strings into datetime objects and then filter those that fall on weekends.
Go to:
Previous: Write a Python program to display a simple, formatted calendar of a given year and month.
Next: Write a Python program to get a list of dates between two dates.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.