Python: Print next 5 days starting from today
9. Next 5 Days Printer
Write a Python program to print the next 5 days starting today.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Get the current date and time and assign it to the variable 'base' using datetime.datetime.today()
base = datetime.datetime.today()
# Iterate over a range of 5 days starting from 0
for x in range(0, 5):
# Print the date and time by adding 'x' days to the base date using datetime.timedelta(days=x)
print(base + datetime.timedelta(days=x))
Output:
2017-05-06 12:27:53.632939 2017-05-07 12:27:53.632939 2017-05-08 12:27:53.632939 2017-05-09 12:27:53.632939 2017-05-10 12:27:53.632939
Explanation:
In the exercise above,
- The code imports the "datetime" module, which provides functionalities to work with dates and times.
- Get the base date and time:
- It gets the current date and time using datetime.datetime.today() and assigns it to the variable 'base'.
- Looping over days:
- It iterates over a range of 5 days starting from 0 using a "for" loop with the "range(0, 5)" function call.
- In each iteration, it prints the date and time by adding the current iteration value (representing the number of days to add) to the base date using "base + datetime.timedelta(days=x)".
- This "timedelta" object adds 'x' days to the base date, effectively giving the date 'x' days in the future from the base date.
- Finally it prints the resulting date and time for each iteration, representing the current date and time plus 'x' days.
Flowchart:
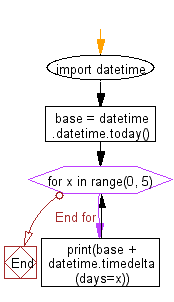
For more Practice: Solve these Related Problems:
- Write a Python program to generate and print a list of the dates for the next five business days, excluding weekends.
- Write a Python script to print the next five dates starting today and then highlight which dates fall on a weekend.
- Write a Python program to output the next five days’ dates and also display the corresponding day names.
- Write a Python function to generate the dates for the next five days and then format each date as "Day, DD Month YYYY".
Go to:
Previous: Write a Python program to convert the date to datetime (midnight of the date).
Next: Write a Python program to add 5 seconds with the current time.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.