Creating a Blue rectangle on a Tkinter canvas widget
Write a Python program to create a Tkinter window with a canvas widget that displays a blue rectangle.
Sample Solution:
Python Code:
import tkinter as tk
# Create the main Tkinter window
root = tk.Tk()
root.title("Blue Rectangle")
# Create a Canvas widget
canvas = tk.Canvas(root, width=300, height=200, bg="white")
canvas.pack()
# Draw a blue rectangle on the Canvas
rectangle = canvas.create_rectangle(50, 50, 250, 150, fill="blue")
# Start the Tkinter event loop
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" for creating GUI components.
- Create the main Tkinter window using tk.Tk() and set its title to "Blue Rectangle."
- Create a Canvas widget using tk.Canvas(), specifying its width, height, and background color.
- Draw a blue rectangle on the "Canvas" using the "canvas.create_rectangle()" method. The coordinates (50, 50) and (250, 150) specify the top-left and bottom-right corners of the rectangle, and fill="blue" sets the fill color to blue.
- The main event loop, root.mainloop(), starts the Tkinter application.
Output:
Flowchart:
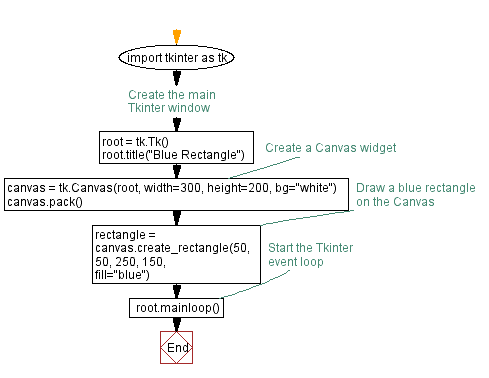
Go to:
Previous: Python Tkinter Canvas and Graphics Home.
Next: Building a paint application with Python and Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.