Create a contact information form with Python Tkinter
Python tkinter layout management: Exercise-9 with Solution
Write a Python program that implements a contact information form with labels and Entry widgets. Organize them within a Frame widget using the Place geometry manager.
Sample Solution:
Python Code:
import tkinter as tk
class ContactForm:
def __init__(self, parent):
self.parent = parent
self.parent.title("Contact Information Form")
# Create a Frame to hold the form elements
self.form_frame = tk.Frame(parent, padx=20, pady=20)
self.form_frame.pack()
# Create labels and Entry widgets for the contact form
self.name_label = tk.Label(self.form_frame, text="Name:")
self.name_label.grid(row=0, column=0, sticky="w")
self.name_entry = tk.Entry(self.form_frame)
self.name_entry.grid(row=0, column=1)
self.email_label = tk.Label(self.form_frame, text="Email:")
self.email_label.grid(row=1, column=0, sticky="w")
self.email_entry = tk.Entry(self.form_frame)
self.email_entry.grid(row=1, column=1)
self.phone_label = tk.Label(self.form_frame, text="Phone:")
self.phone_label.grid(row=2, column=0, sticky="w")
self.phone_entry = tk.Entry(self.form_frame)
self.phone_entry.grid(row=2, column=1)
# Create a Submit button
self.submit_button = tk.Button(self.form_frame, text="Submit", command=self.submit_form)
self.submit_button.grid(row=3, columnspan=2, pady=10)
def submit_form(self):
# Retrieve and display the submitted data
name = self.name_entry.get()
email = self.email_entry.get()
phone = self.phone_entry.get()
# Display the submitted data in a message box
message = f"Name: {name}\nEmail: {email}\nPhone: {phone}"
tk.messagebox.showinfo("Submitted Information", message)
if __name__ == "__main__":
parent = tk.Tk()
app = ContactForm(parent)
parent.mainloop()
Explanation:
In the exercise above -
- We create a class "ContactForm" that encapsulates the contact form application.
- The name, email, and phone fields are created and placed within a frame named 'form_frame' using the "Place geometry manager".
- A "Submit" button is created, and its command is set to the 'submit_form' method.
- By using tk.messagebox.showinfo, the submit_form method displays the values entered in Entry widgets in a message box.
- The program starts the Tkinter main loop to run the application.
Sample Output:
Flowchart:
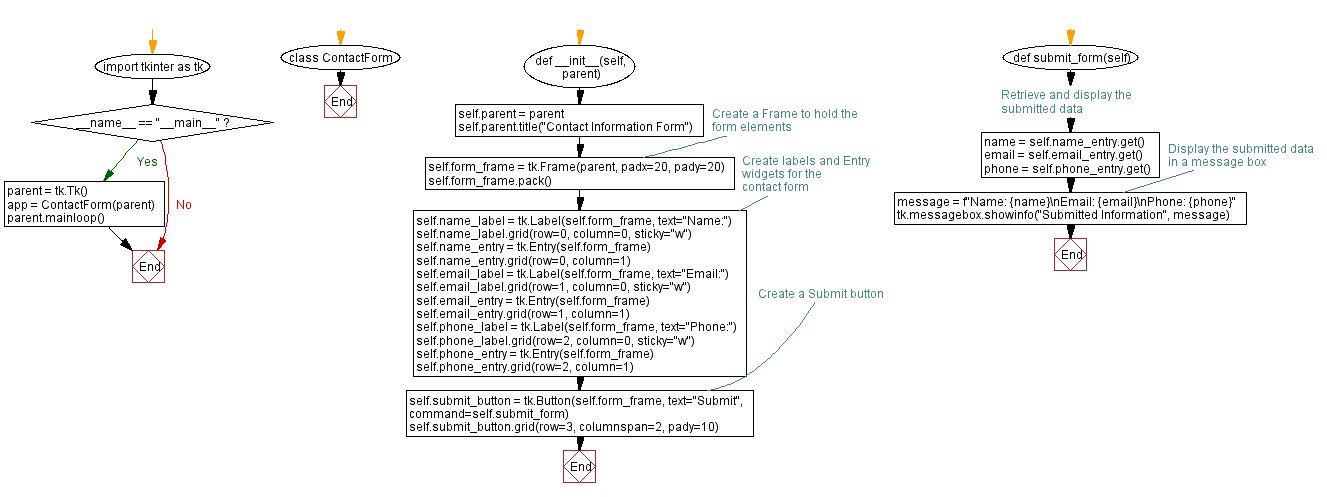
Python Code Editor:
Previous: Create a basic calendar application with Python Tkinter.
Next: Create a dashboard with Python and Tkinter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics