C Exercises: Double its value and replace the next number with 0 if current and next value are same and shift all 0's to the end
106. Array Transformation: Double Value & Zero Shift
Write a C program to convert an array in such a way that it doubles its value. This will replace the next element with 0 if the current and next elements are the same. This program will rearrange the array so that all 0's are moved to the end.
Expected Output:
The given array is: 0 3 3 3 0 0 7 7 0 9
The new array is: 6 3 14 9 0 0 0 0 0 0
The task is to write a C program that converts an array such that each element doubles its value. If an element and its next element are the same, the next element is replaced with 0. After these transformations, the program should rearrange the array so that all 0s are moved to the end. The output should display the modified array according to these transformations.
Sample Solution:
C Code:
#include<stdio.h>
// Function to move all non-zero elements to the beginning of the array
void ZerosAtEnd(int arr1[], int n)
{
int ctr = 0;
for (int i = 0; i < n; i++)
if (arr1[i] != 0)
arr1[ctr++] = arr1[i]; // Shift non-zero elements to the front
while (ctr < n)
arr1[ctr++] = 0; // Fill remaining positions with zeros
}
// Function to update the array by merging consecutive identical non-zero elements and moving zeros to the end
void updateArrayRearrange(int arr1[], int n)
{
if (n == 1)
return; // If the array has only one element, return without any change
for (int i = 0; i < n - 1; i++)
{
if ((arr1[i] != 0) && (arr1[i] == arr1[i + 1]))
{
arr1[i] = 2 * arr1[i]; // Merge consecutive identical elements by doubling the first element
arr1[i + 1] = 0; // Set the next element as 0
i++; // Skip the next element as it's already processed
}
}
ZerosAtEnd(arr1, n); // Move all zeros to the end of the array
}
// Function to print the array
void ArrayPrinting(int arr1[], int n)
{
for (int i = 0; i < n; i++)
printf("%d ",arr1[i]); // Print each element of the array
}
// Main function
int main()
{
int arr1[] = { 0, 3, 3, 3, 0, 0, 7, 7, 0, 9 };
int n = sizeof(arr1) / sizeof(arr1[0]);
printf("The given array is: ");
ArrayPrinting(arr1, n); // Display the initial array
updateArrayRearrange(arr1, n); // Update the array by merging consecutive identical elements and moving zeros
printf("\nThe new array is: ");
ArrayPrinting(arr1, n); // Display the modified array
return 0;
}
Output:
The given array is: 0 3 3 3 0 0 7 7 0 9 The new array is: 6 3 14 9 0 0 0 0 0 0
Flowchart 1:
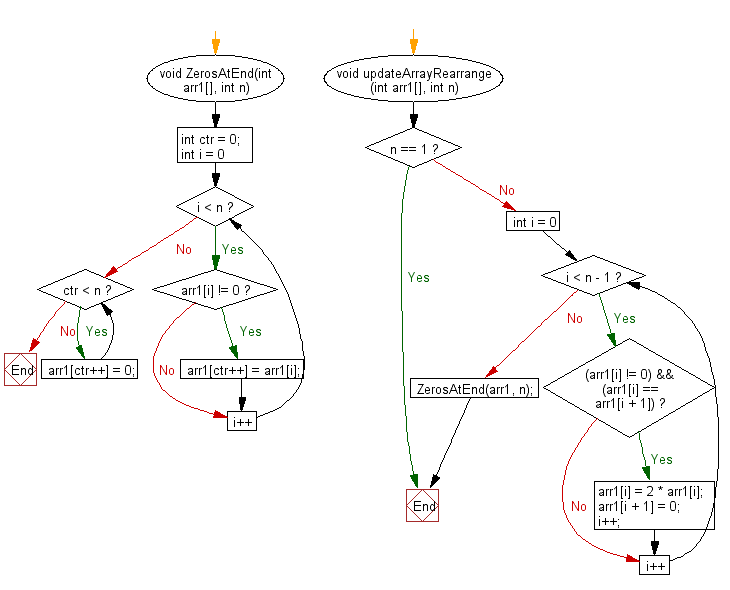
Flowchart 2:
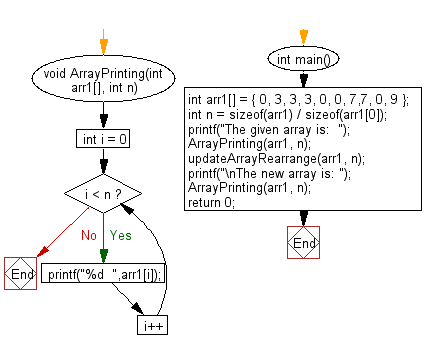
For more Practice: Solve these Related Problems:
- Write a C program to transform an array by doubling each element and then shifting zeros to the end.
- Write a C program to update an array such that if consecutive elements are equal, replace the first with their sum and the second with zero, then move zeros to the end.
- Write a C program to process an array and, for each pair of equal adjacent numbers, double the first and set the second to zero before segregating zeros.
- Write a C program to rearrange an array so that identical adjacent elements are merged (doubled) and zeros are shifted to the right.
C Programming Code Editor:
Previous C Programming Exercise: Minimum swaps to gather all elements less or equal to k.
Next C Programming Exercise: Concatenate two arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.