C Exercises: Subtraction of two Matrices
C Array: Exercise-20 with Solution
Write a program in C for the subtraction of two matrices.
The task is to write a C program that subtracts two square matrices of the same size. The program prompts the user to input the size of the matrices (less than 5), elements for each matrix, and then calculates the difference between the matrices. It displays the original matrices and their subtraction result as output.
Visual Presentation:
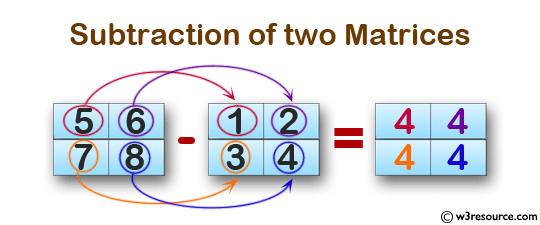
Sample Solution:
C Code:
#include <stdio.h>
void main() {
int arr1[50][50], brr1[50][50], crr1[50][50], i, j, n;
// Prompt user for input
printf("\n\nSubtraction of two Matrices :\n");
printf("------------------------------\n");
printf("Input the size of the square matrix (less than 5): ");
scanf("%d", &n);
// Input elements for the first matrix
printf("Input elements in the first matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Input elements for the second matrix
printf("Input elements in the second matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &brr1[i][j]);
}
}
// Display the first matrix
printf("\nThe First matrix is :\n");
for (i = 0; i < n; i++) {
printf("\n");
for (j = 0; j < n; j++)
printf("%d\t", arr1[i][j]);
}
// Display the second matrix
printf("\nThe Second matrix is :\n");
for (i = 0; i < n; i++) {
printf("\n");
for (j = 0; j < n; j++)
printf("%d\t", brr1[i][j]);
}
// Calculate the subtraction of the matrices
for (i = 0; i < n; i++)
for (j = 0; j < n; j++)
crr1[i][j] = arr1[i][j] - brr1[i][j];
// Display the subtraction of two matrices
printf("\nThe Subtraction of two matrix is : \n");
for (i = 0; i < n; i++) {
printf("\n");
for (j = 0; j < n; j++)
printf("%d\t", crr1[i][j]);
}
printf("\n\n");
}
Sample Output:
Subtraction of two Matrices : ------------------------------ Input the size of the square matrix (less than 5): 2 Input elements in the first matrix : element - [0],[0] : 5 element - [0],[1] : 6 element - [1],[0] : 7 element - [1],[1] : 8 Input elements in the second matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 The First matrix is : 5 6 7 8 The Second matrix is : 1 2 3 4 The Subtraction of two matrix is : 4 4 4 4
Flowchart:
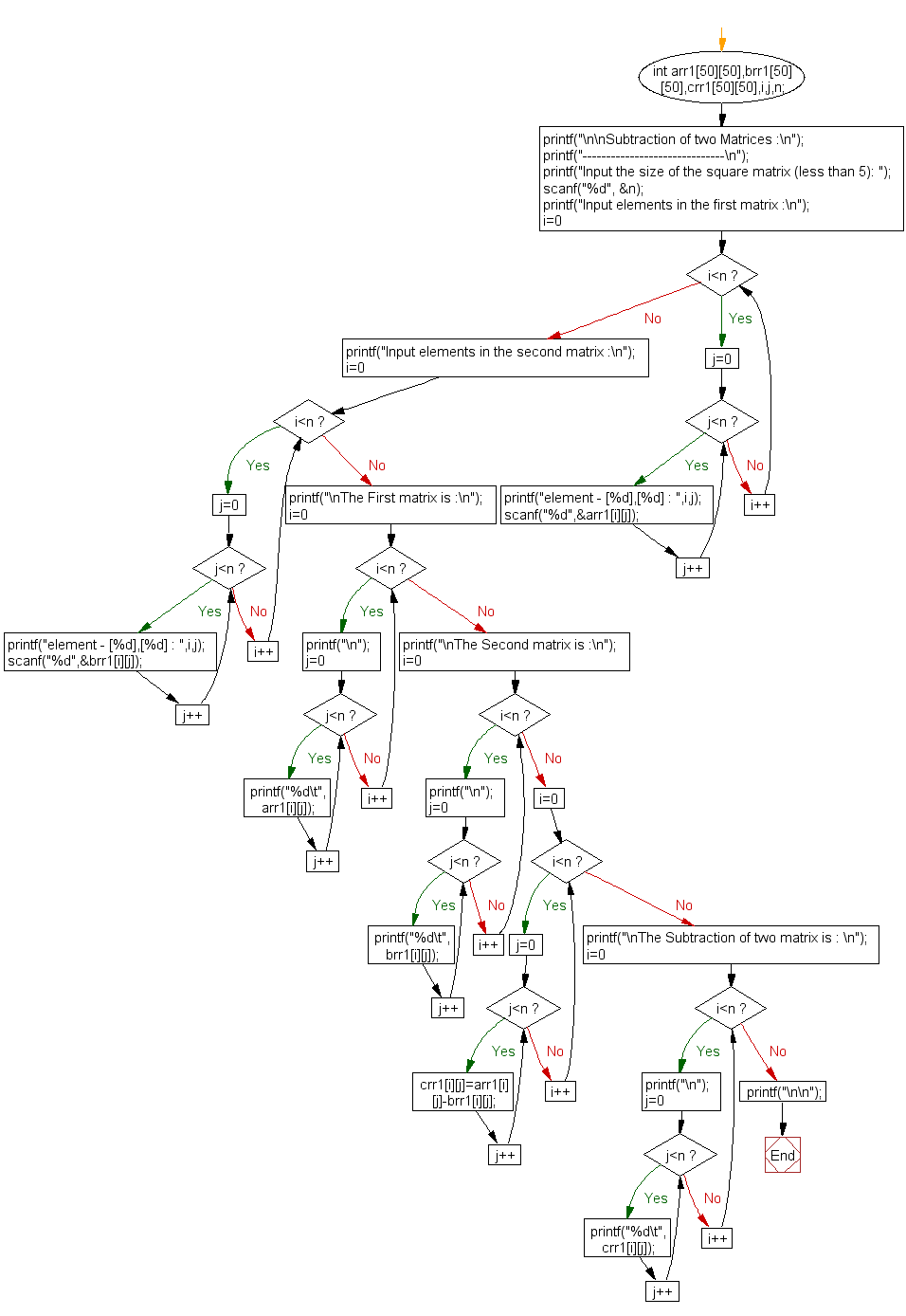
C Programming Code Editor:
Previous: Write a program in C for addition of two Matrices of same size.
Next: Write a program in C for multiplication of two square Matrices.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/array/c-array-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics