C Exercises: Find if a given integer x appears more than n/2 times in a sorted array of n integers
48. Majority Element Occurrence (> n/2)
Write a program in C to find out if a given integer x appears more than n/2 times in a sorted array of n integers.
The program checks if a specified integer appears more than half the times in a sorted array. It counts the occurrences of the integer and compares it to half the length of the array, printing the result.
Visual Presentation:
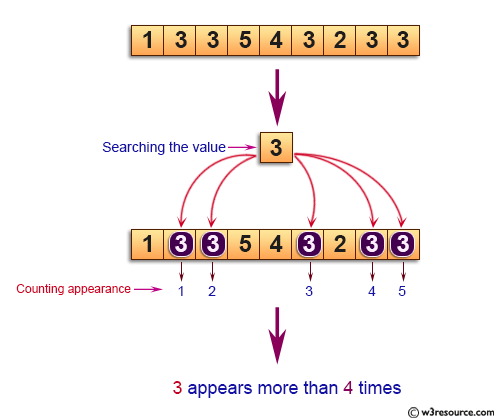
Sample Solution:
C Code:
# include <stdio.h>
# include <stdbool.h>
// Function to check if 'x' appears more than n/2 times in arr1[]
bool ChkMajority(int arr1[], int arr_size, int x)
{
int i;
// Check if the last index is even or odd to decide the range
int last_index = arr_size % 2 ? (arr_size / 2 + 1) : (arr_size / 2);
for (i = 0; i < last_index; i++)
{
// Check if the element at current index and the corresponding element after mid point equals 'x'
if (arr1[i] == x && arr1[i + arr_size / 2] == x)
return true; // Return true if 'x' appears more than n/2 times
}
return false; // Return false if 'x' does not appear more than n/2 times
}
int main()
{
int arr1[] = {1, 3, 3, 5, 4, 3, 2, 3, 3};
int arr_size = sizeof(arr1) / sizeof(arr1[0]);
int x = 3, i;
// Print the given array
printf("The given array is : ");
for (i = 0; i < arr_size; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
printf("The given value is : %d\n", x);
// Check if 'x' appears more than n/2 times in the array
if (ChkMajority(arr1, arr_size, x))
printf("%d appears more than %d times in the given array[]", x, arr_size / 2);
else
printf("%d does not appear more than %d times in the given array[]", x, arr_size / 2);
return 0;
}
Sample Output:
The given array is : 1 3 3 5 4 3 2 3 3 The given value is : 3 3 appears more than 4 times in the given array[]
Flowchart:
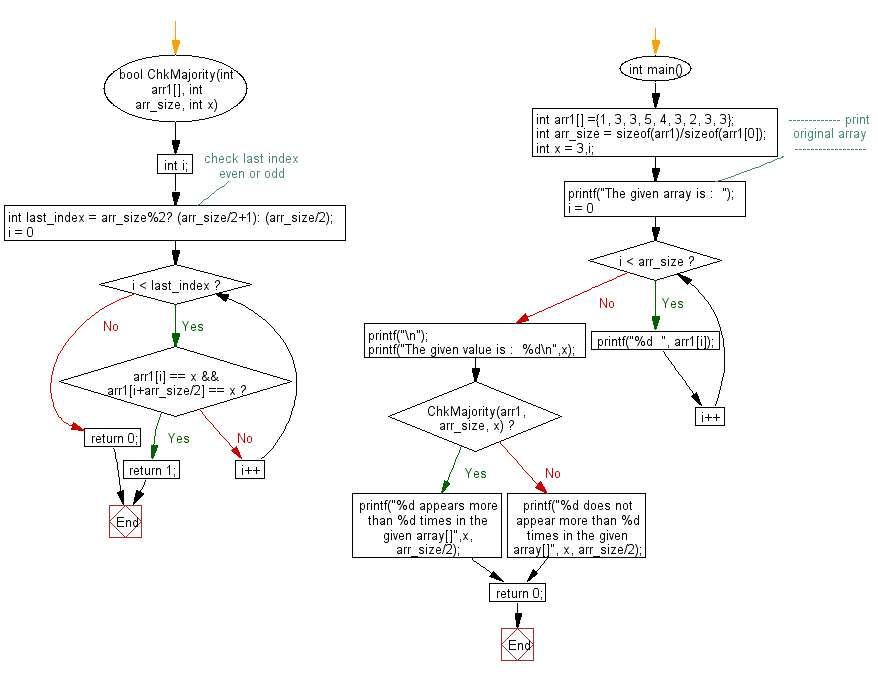
For more Practice: Solve these Related Problems:
- Write a C program to check if a given integer appears more than n/2 times using the Boyer-Moore algorithm.
- Write a C program to count occurrences of each element and then determine if any appears more than n/2 times.
- Write a C program to identify a majority element using a hash table approach.
- Write a C program to verify majority occurrence and output the count along with the element.
C Programming Code Editor:
Previous: Write a program in C to find a subarray with given sum from the given array.
Next: Write a program in C to find majority element of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.