C Exercises: Find majority element of an array
49. Majority Element (General)
Write a program in C to find the majority element of an array.
This task involves writing a C program to find and display the majority element in an array, which is the element that appears more than half the time. The program will take the array as input, analyze the frequency of each element, and then determine and print the majority element.
Visual Presentation:
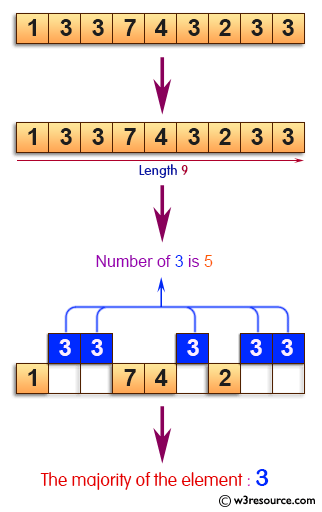
Sample Solution:
C Code:
# include <stdio.h>
// Function to find the majority element in the array
void findMajorityElement(int *arr1, int arr_size)
{
int i, mIndex = 0, ctr = 1;
// Finding the potential majority element
for(i = 1; i < arr_size; i++)
{
if(arr1[mIndex] == arr1[i])
ctr++;
else
ctr--;
// Reset the candidate majority element when the counter reaches 0
if(ctr == 0)
{
mIndex = i;
ctr = 1;
}
}
ctr = 0;
// Count the frequency of the potential majority element
for (i = 0; i < arr_size; i++)
{
if(arr1[i] == arr1[mIndex])
ctr++;
}
// Check if the frequency of the potential majority element is greater than arr_size/2
if(ctr > (arr_size/2))
printf("The majority of the Element : %d\n", arr1[mIndex]);
else
printf("No majority element found in the array.\n");
}
int main()
{
int i;
int arr1[] = {1, 3, 3, 7, 4, 3, 2, 3, 3};
int ctr = sizeof(arr1) / sizeof(arr1[0]);
// Print the given array
printf("The given array is : ");
for(i = 0; i < ctr; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
// Find the majority element in the array
findMajorityElement(arr1, ctr);
return 0;
}
Sample Output:
The given array is : 1 3 3 7 4 3 2 3 3 The majority of the Element : 3
Flowchart:
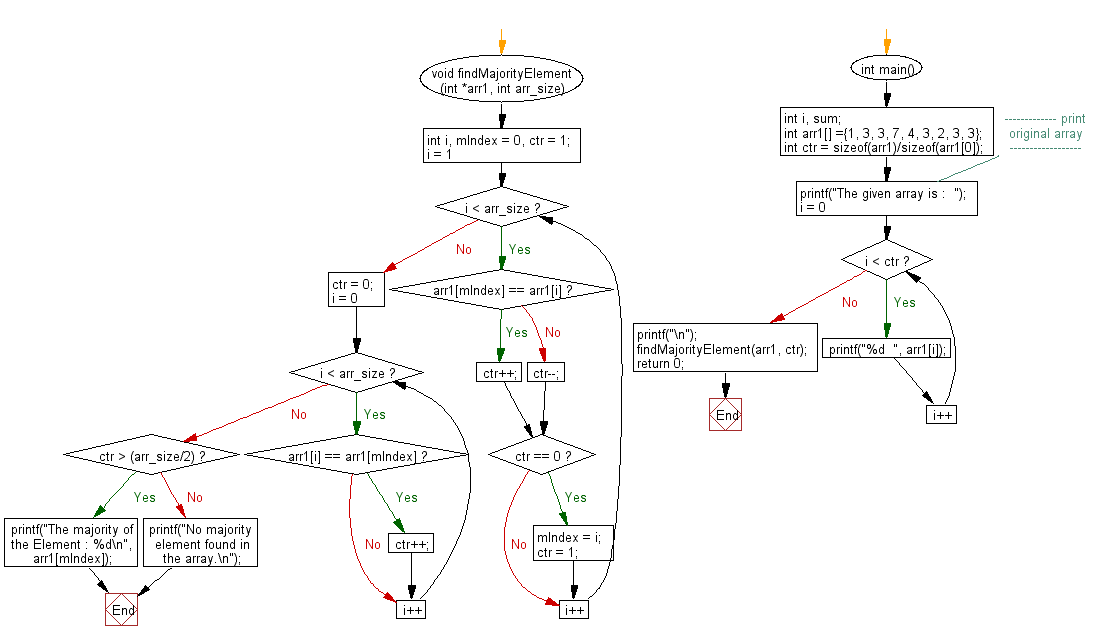
For more Practice: Solve these Related Problems:
- Write a C program to find the majority element in an array and display its frequency.
- Write a C program to determine the majority element by sorting the array first and then scanning.
- Write a C program to identify the majority element using recursion to divide the array.
- Write a C program to check for a majority element and then compare it with the sum of all other elements.
C Programming Code Editor:
Previous: Write a program in C to find if a given integer x appears more than n/2 times in a sorted array of n integers.
Next: Write a program in C to print a matrix in spiral form.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.