C Exercises: Find the maximum element in an array which is first increasing and then decreasing
C Array: Exercise-87 with Solution
Write a program in C to find the maximum element in an array that is first increasing and then decreasing.
Expected Output:
The given array is:
2 7 12 25 4 57 27 44
The maximum element which is increasing then decreasing is: 57
The task requires writing a C program to find the maximum element in an array where the elements first increase and then decrease. The program should iterate through the array to identify the peak element that satisfies this condition. The output will display the array and the maximum element that is part of the increasing-then-decreasing sequence.
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the maximum element in an array that increases then decreases
int firstIncreDecre(int arr1[], int n)
{
int max_ele = arr1[0]; // Initialize the maximum element as the first element
// Loop through the array to find the maximum element
for (int i = 1; i < n; i++)
{
if (arr1[i] > max_ele)
max_ele = arr1[i]; // Update max_ele if a greater element is found
}
return max_ele; // Return the maximum element
}
int main()
{
int arr1[] = {2, 7, 12, 25, 4, 57, 27, 44}; // Fill the array by increasing and decreasing elements
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
// Print the original array
printf("The given array is: \n");
for (i = 0; i < n; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
// Find and print the maximum element which increases then decreases
printf("The maximum element which is increasing then decreasing is: %d ", firstIncreDecre(arr1, n));
return 0;
}
Output:
The given array is: 2 7 12 25 4 57 27 44 The maximum element which is increasing then decreasing is: 57
Flowchart:
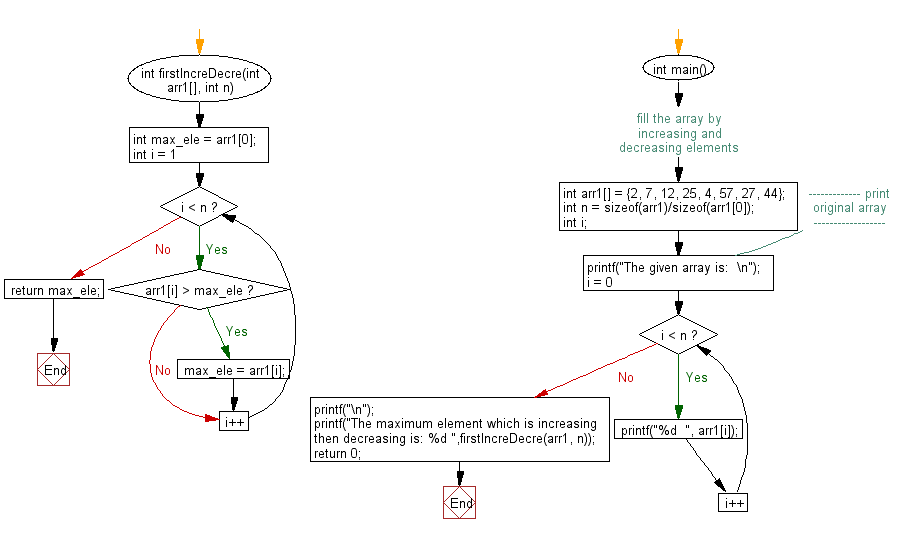
C Programming Code Editor:
Previous: Write a program in C to find the equilibrium index of an array.
Next: Write a program in C to find the maximum n – m.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics