C Exercises: Check which number nearest to the value 100 among two given integers
C-programming basic algorithm: Exercise-10 with Solution
Write a C program to check which number is nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Declare the function 'test' with two integer parameters
int test(int x, int y);
int main(void)
{
// Call the function 'test' with arguments 78 and 95, and print the result
printf("%d", test(78, 95));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 95 and 95, and print the result
printf("%d", test(95, 95));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 99 and 70, and print the result
printf("%d", test(99, 70));
}
// Function definition for 'test'
int test(int x, int y)
{
int n = 100; // Define a variable 'n' and assign it the value 100
int val = abs(x - n); // Calculate the absolute difference between 'x' and 'n' and store it in 'val'
int val2 = abs(y - n); // Calculate the absolute difference between 'y' and 'n' and store it in 'val2'
// Check if 'val' is equal to 'val2'. If true, return 0. Otherwise, return 'x' if 'val' is less than 'val2', otherwise return 'y'.
return val == val2 ? 0 : (val < val2 ? x : y);
}
Sample Output:
95 0 99
Explanation:
int test(int x, int y) { int n = 100; int val = abs(x - n); int val2 = abs(y - n); return val == val2 ? 0 : (val < val2 ? x : y); }
The above function initializes an integer ‘n’ with a value of 100. Then it initializes two integer variables val and val2 with the absolute difference between ‘x’ and ‘n’ and ‘y’ and ‘n’, respectively.
Next, the function checks if ‘val’ is equal to ‘val2’. If it is, then the function returns 0. Otherwise, the function checks which of the two values ‘val’ and ‘val2’ is smaller. If ‘val’ is smaller, then the function returns ‘x’. If ‘val2’ is smaller, then the function returns ‘y’.
Time complexity and space complexity:
Time complexity: The time complexity of this function is O(1) since the operations performed are simple arithmetic operations and a few conditional statements, and the number of operations is constant regardless of the input size.
Space complexity: The space complexity of this function is O(1) since the function only uses a fixed number of integer variables and does not create any data structures or arrays.
Pictorial Presentation:
Flowchart:
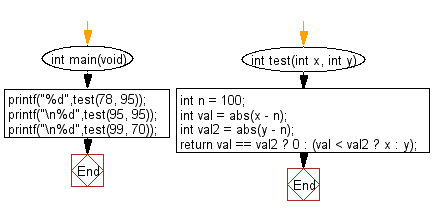
C Programming Code Editor:
Previous: Write a C program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
Next: Write a C program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics