C Exercises: Check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive
C-programming basic algorithm: Exercise-11 with Solution
Write a C program that checks if two given integers are in the range of 40 to 50 inclusive, or if they are both in the range of 50 to 60 inclusive.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Declare the function 'test' with two integer parameters
int test(int x, int y);
int main(void)
{
// Call the function 'test' with arguments 78 and 95, and print the result
printf("%d", test(78, 95));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 25 and 35, and print the result
printf("%d", test(25, 35));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 40 and 50, and print the result
printf("%d", test(40, 50));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 55 and 60, and print the result
printf("%d", test(55, 60));
}
// Function definition for 'test'
int test(int x, int y)
{
// Check if both 'x' and 'y' are within the ranges [40, 50] and [50, 60] respectively. If true, return 1. Otherwise, return 0.
return (x >= 40 && x <= 50 && y >= 40 && y <= 50) || (x >= 50 && x <= 60 && y >= 50 && y <= 60);
}
Sample Output:
0 0 1 1
Explanation:
int test(int x, int y) { return (x >= 40 && x <= 50 && y >= 40 && y <= 50) || (x >= 50 && x <= 60 && y >= 50 && y <= 60); }
The said function takes two integer inputs ‘x’ and ‘y’ and checks whether both the inputs fall in two different ranges or not. The first range is between 40 and 50 (inclusive), and the second range is between 50 and 60 (inclusive).
Time complexity and space complexity:
Time Complexity of the function is O(1) as it involves only a few comparisons.
The Space Complexity of the function is O(1) as it uses only a few constant variables.
Pictorial Presentation:
Flowchart:
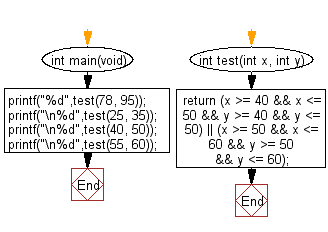
C Programming Code Editor:
Previous: Write a C program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Next: Write a C program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics