C Exercises: Find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range
12. Range 20 to 30 with Larger Value
Write a C program that takes two positive integer values as input and checks if either of them is in the range of 20 to 30 (inclusive). If at least one number falls in this range, the program returns the larger value. Otherwise, it returns 0.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with two integer parameters
int test(int x, int y);
int main(void) {
// Call the function 'test' with arguments 78 and 95, and print the result
printf("%d", test(78, 95));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 20 and 30, and print the result
printf("%d", test(20, 30));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 21 and 25, and print the result
printf("%d", test(21, 25));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 28 and 28, and print the result
printf("%d", test(28, 28));
}
// Function definition for 'test'
int test(int x, int y) {
if (x >= 20 && x <= 30 && y >= 20 && y <= 30) { // Check if both 'x' and 'y' are within the range [20, 30]
if (x >= y) { // If 'x' is greater than or equal to 'y'
return x; // Return 'x'
} else {
return y; // Return 'y'
}
} else if (x >= 20 && x <= 30) { // If only 'x' is within the range [20, 30]
return x; // Return 'x'
} else if (y >= 20 && y <= 30) { // If only 'y' is within the range [20, 30]
return y; // Return 'y'
} else { // If neither 'x' nor 'y' is within the range [20, 30]
return 0; // Return 0
}
}
Sample Output:
0 30 25 28
Explanation:
int test(int x, int y) { if (x >= 20 && x <= 30 && y >= 20 && y <= 30) { if (x >= y) { return x; } else { return y; } } else if (x >= 20 && x <= 30) { return x; } else if (y >= 20 && y <= 30) { return y; } else { return 0; } }
The above function takes two integer inputs x and y and returns the maximum value among them based on some conditions. The conditions are as follows:
- If both x and y are between 20 and 30 (inclusive), then return the maximum value between x and y.
- If x is between 20 and 30 (inclusive) but y is not, then return x.
- If y is between 20 and 30 (inclusive) but x is not, then return y.
- Otherwise, return 0.
Time complexity and space complexity:
The time complexity of this function is O(1), since the function only performs a constant number of comparisons and returns a result based on the conditions.
The space complexity of this function is also O(1), since it only uses a constant amount of memory to store the input variables and local variables.
Pictorial Presentation:
Flowchart:
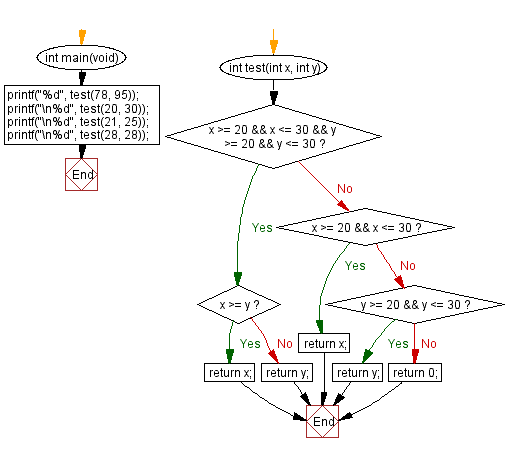
For more Practice: Solve these Related Problems:
- Write a C program that takes two positive integers and returns the smaller one if at least one lies within 25 to 35.
- Write a C program that checks if one of two numbers is in the range 15 to 25 and returns that number doubled.
- Write a C program that returns the maximum of two numbers only if both are within the range 20 to 40, otherwise return their sum.
- Write a C program to check two integers; if one falls between 10 and 20, return the difference between them; otherwise, return 0.
Go to:
PREV : Dual Range Check (40-50 and 50-60).
NEXT : Same Last Digit Check.
C Programming Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.