C Exercises: Check whether a given array of integers of length 2, does not contain 15 or 20
42. Array of Two: Exclude 15 and 20
Write a C program to check if an array of integers with length 2 does not contain 15 or 20.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[]);
int main(void){
// Declaration and initialization of variables
int arr_size;
int array1[] = {12, 20};
// Printing the result of calling 'test' function with different arrays
printf("%d", test(array1));
int array2[] = {14, 15};
printf("\n%d", test(array2));
int array3[] = {11, 21};
printf("\n%d", test(array3));
}
// Definition of the 'test' function
int test(int nums[])
{
// Checking if none of the elements in the array are equal to 12 or 15
return nums[0] != 12 && nums[0] != 15 && nums[1] != 12 && nums[1] != 15;
}
Sample Output:
0 0 1
Pictorial Presentation:
Flowchart:
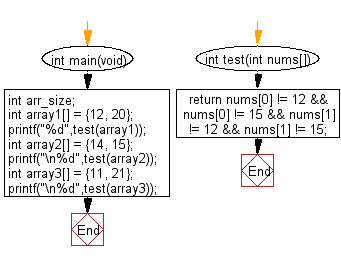
For more Practice: Solve these Related Problems:
- Write a C program to ensure an array of two integers does not contain 10 or 30.
- Write a C program to check if an array of length 2 has no even numbers.
- Write a C program to determine if an array of two numbers excludes both 7 and 8.
- Write a C program to verify that an array of two integers does not contain any multiples of 3.
C Programming Code Editor:
Previous: Write a C program to check whether a given array of integers of length 2, contains 15 or 20.
Next: Write a C program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.