C Exercises: Check a given array of integers and return true if the array contains 10 or 20 twice
43. Array Contains Double 10 or 20
Write a C program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int size);
int main(void){
// Declaration and initialization of variables
int arr_size;
int array1[] = {12, 20};
// Calculating the size of the array
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of calling 'test' function with the first array
printf("%d", test(array1, arr_size));
int array2[] = {20, 20};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of calling 'test' function with the second array
printf("\n%d", test(array2, arr_size));
int array3[] = {10};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of calling 'test' function with the third array
printf("\n%d", test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int size)
{
// Checking if the size is 2 and if the elements meet specific conditions
return size == 2 &&
((nums[0] == 10 && nums[1] == 10) || (nums[0] == 20 && nums[1] == 20));
}
Sample Output:
0 1 0
Pictorial Presentation:
Flowchart:
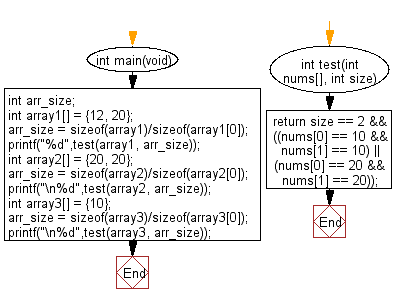
For more Practice: Solve these Related Problems:
- Write a C program to check if an array of at most 2 integers contains two occurrences of 5 or 15.
- Write a C program to determine if an array of two elements contains duplicate numbers that are either 25 or 35.
- Write a C program to verify if an array of up to two integers contains duplicate even numbers.
- Write a C program to check if an array with a maximum length of 2 has two instances of any number divisible by 5.
C Programming Code Editor:
Previous: Write a C program to check if a given array of integers and length 2, does not contain 15 or 20.
Next: Write a C program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.