C Exercises: Check a given array of integers of length 3 and create a new array
44. Modify Array: 5 Followed by 7 to 5 and 1
Write a C program to check a given array of integers of length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration and initialization of variables
int arr_size;
int array1[] = {1, 5, 7};
// Calculating the size of the array
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing elements in the original array
printf("Elements in original array are: ");
print_array(array1, arr_size);
// Loop through the array to check for specific condition and modify elements
for (int i = 0; i < arr_size - 1; i++)
{
if (array1[i] == 5 && array1[i + 1] == 7)
array1[i + 1] = 1;
}
// Printing elements in the modified array
printf("Elements in new array are: ");
print_array(array1, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array are: 1, 5, 7 Elements in new array are: 1, 5, 1
Pictorial Presentation:
Flowchart:
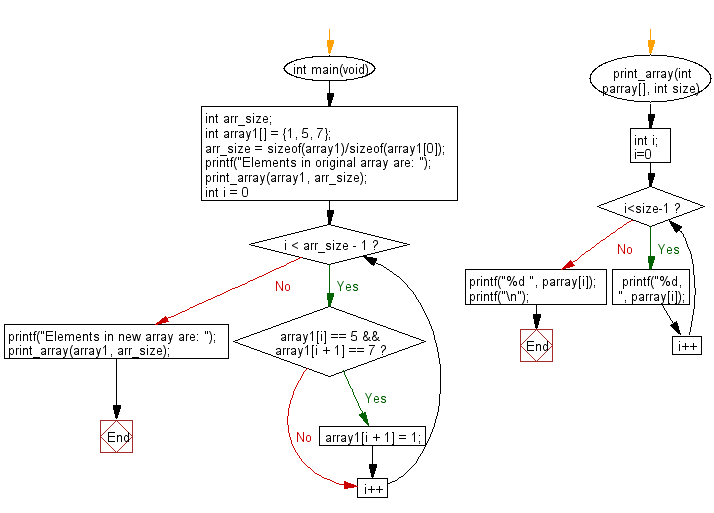
For more Practice: Solve these Related Problems:
- Write a C program to change every occurrence of 7 preceded by 3 to 0 in an array of length 3.
- Write a C program to replace the number following 4 with 9 in a 3-element array.
- Write a C program to modify a 3-element array so that if a 6 is immediately followed by an 8, replace 8 with 2.
- Write a C program to check a 3-element array and if the sequence 2, 4 appears, replace 4 with 0.
C Programming Code Editor:
Previous: Write a C program to check a given array of integers and return true if the array conains 10 or 20 twice.The length of the array will be 0, 1, or 2.
Next: Write a C program to compute the sum of the two given arrays of integers of length 3 and find the array which has the largest sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.