C Exercises: Count number of even elements in a given array of integers
51. Count Even Elements in Array
Write a C program to count the even number of elements in a given array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 2, 5, 7, 9, 10, 12};
// Calculating the size of the array 'array1'
int arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for the array 'array1'
printf("%d",test(array1, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Declaration of a variable 'evens' to count even numbers
int evens = 0;
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is even
if (nums[i] % 2 == 0) evens++;
}
// Returning the count of even numbers
return evens;
}
Sample Output:
3
Pictorial Presentation:
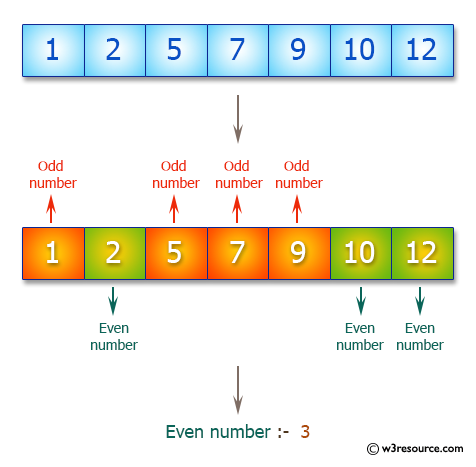
Flowchart:
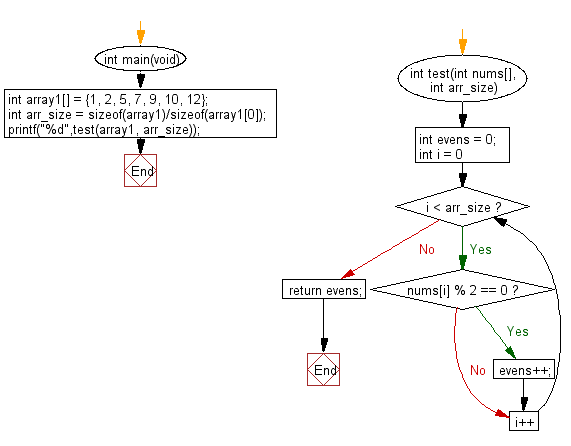
For more Practice: Solve these Related Problems:
- Write a C program to count the odd numbers in an array of integers.
- Write a C program to count how many prime numbers exist in a given array.
- Write a C program to count the number of negative elements in an integer array.
- Write a C program to count the frequency of a specific integer in an array.
C Programming Code Editor:
Previous: Write a C program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
Next: Write a C program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.