C Exercises: Check whether a given array of integers contains 5 next to a 5 somewhere
54. Check for Adjacent 5's in Array
Write a C program to check whether a given array of integers contains 5 next to a 5 somewhere.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
// Declaration and initialization of variables
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 5, 6, 9, 10, 17};
// Calculating the size of the array 'array1'
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {1, 5, 5, 9, 10, 17};
// Calculating the size of the array 'array2'
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {1, 5, 5, 9, 10, 17, 5, 5};
// Calculating the size of the array 'array3'
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Looping through the elements of the array
for (int i = 0; i < arr_size - 1; i++)
{
// Checking if the current element and the next element are both 5
if (nums[i] == 5 && nums[i] == nums[i + 1])
{
return 1; // If condition met, return 1 (true)
}
}
return 0; // If condition not met for any pair, return 0 (false)
}
Sample Output:
0 1 1
Pictorial Presentation:
Flowchart:
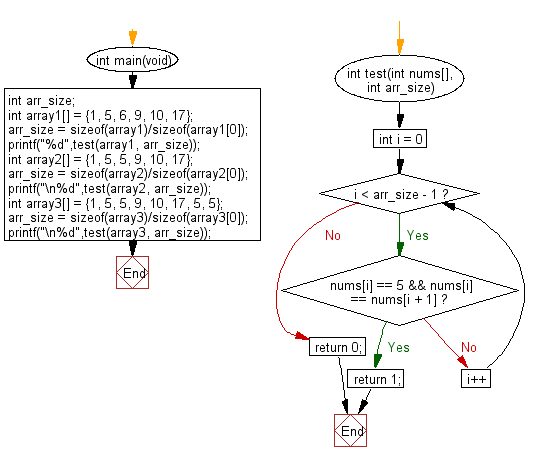
For more Practice: Solve these Related Problems:
- Write a C program to verify if an array contains adjacent pairs of even numbers.
- Write a C program to check if an array has two consecutive numbers that are both prime.
- Write a C program to detect if an array contains two consecutive identical numbers, regardless of their value.
- Write a C program to check if an array contains a 7 immediately followed by another 7.
C Programming Code Editor:
Previous: Write a C program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6. Return 0 if the given array has no integer.
Next: Write a C program to check whether a given array of integers contains 5's and 7's.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.