C Exercises: Check if the sum of all 5' in the array exactly 15 in a given array of integers
C-programming basic algorithm: Exercise-56 with Solution
Write a C program to check if the sum of all 5's in the array is exactly 15 in a given array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 5, 6, 9, 10, 17};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {1, 5, 5, 5, 10, 17};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {1, 1, 5, 5, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
int sum = 0;
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is equal to 5
if (nums[i] == 5)
{
sum += 5; // If condition met, add 5 to 'sum'
}
}
return sum == 15; // Return whether 'sum' is equal to 15
}
Sample Output:
0 1 0
Pictorial Presentation:
Flowchart:
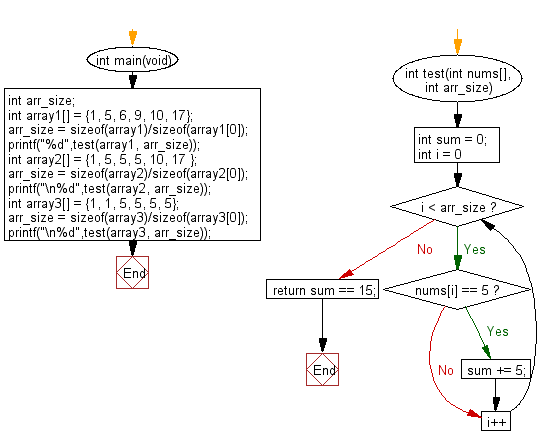
C Programming Code Editor:
Previous: Write a C program to check whether a given array of integers contains 5's and 7's.
Next: Write a C program to check if the number of 3's is greater than the number of 5's.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-56.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics