C Exercises: Check if a given array of integers contains no 3 or a 5
59. Array Contains No 3 or 5
Write a C program to check if a given array of integers contains no 3 or 5.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 5, 5, 5, 5};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {3, 3, 3, 3 };
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {3, 3, 3, 5, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
// Declaration and initialization of an integer array 'array4'
int array4[] = {1, 6, 8, 10};
arr_size = sizeof(array4)/sizeof(array4[0]);
// Printing the result of the 'test' function for 'array4'
printf("\n%d",test(array4, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
int three = 0, five = 0;
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
// Checking if the current element is 3
if (nums[i] == 3)
{
three++;
}
// Checking if the current element is 5
if (nums[i] == 5)
{
five++;
}
// If both 3 and 5 are found, return 0 (false)
if (three && five)
{
return 0;
}
}
return 1; // If no instance of both 3 and 5, return 1 (true)
}
Sample Output:
1 1 0 1
Pictorial Presentation:
Flowchart:
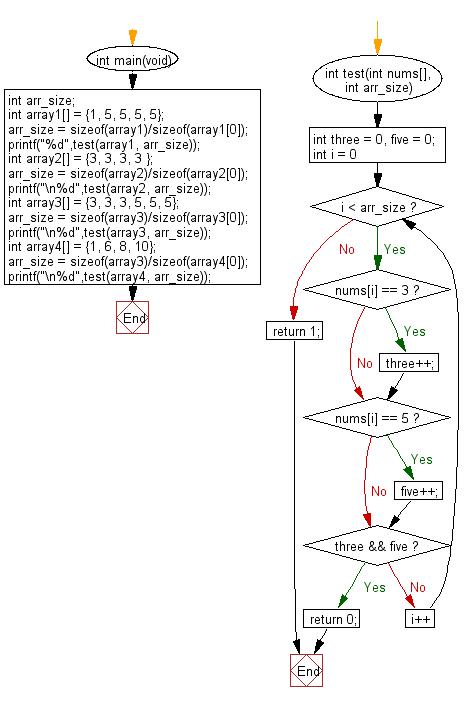
For more Practice: Solve these Related Problems:
- Write a C program to check if an array contains neither 1 nor 2.
- Write a C program to verify that an array does not contain any prime numbers.
- Write a C program to determine if an array lacks both even and odd numbers (i.e., it's empty or only zeros).
- Write a C program to check if an array does not contain any multiples of 7.
C Programming Code Editor:
Previous: Write a C program to check if a given array of integers contains a 3 or a 5.
Next: Write a C program to check if an array of integers contains a 3 next to a 3 or a 5 next to a 5 or both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.