C Exercises: Check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other
63. Check for Adjacent Pair of Even or Odd Numbers
Write a C program to check a given array of integers. The program will return true if the given array contains either 2 even or 2 odd values all next to each other.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int numbers[], int arr_size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {1, 2, 3, 4};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size));
// Declaration and initialization of an integer array 'array2'
int array2[] = {3, 3, 5, 5, 5, 5};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size));
// Declaration and initialization of an integer array 'array3'
int array3[] = {2, 5, 5, 7, 8, 10};
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int numbers[], int arr_size)
{
int tot_odd = 0, tot_even = 0;
// Looping through the elements of the array
for (int i = 0; i < arr_size; i++)
{
if (tot_odd < 2 && tot_even < 2)
{
if (numbers[i] % 2 == 0)
{
tot_even++;
tot_odd = 0;
}
else
{
tot_odd++;
tot_even = 0;
}
}
}
// Returning true if there are exactly 2 consecutive even or odd numbers
return tot_odd == 2 || tot_even == 2;
}
Sample Output:
0 1 1
Pictorial Presentation:
Flowchart:
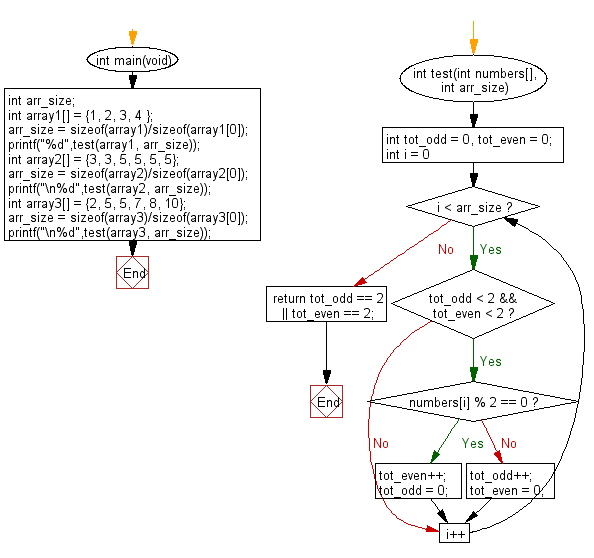
For more Practice: Solve these Related Problems:
- Write a C program to verify if an array contains three consecutive even numbers.
- Write a C program to determine if an array contains a pair of consecutive odd numbers.
- Write a C program to check if an array has at least two consecutive numbers with the same parity.
- Write a C program to check for any sequence of three consecutive numbers that are all even or all odd.
C Programming Code Editor:
Previous: Write a C program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Next: Write a C program to check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.