C Exercises: Check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array
C-programming basic algorithm: Exercise-66 with Solution
Write a C program to check a given array of integers. The program will return true if the specified number of the same elements appears at the start and end of the given array.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int numbers[], int arr_size, int len);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'array1'
int array1[] = {3, 7, 5, 5, 3, 7};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Printing the result of the 'test' function for 'array1'
printf("%d",test(array1, arr_size, 2));
// Declaration and initialization of an integer array 'array2'
int array2[] = {3, 7, 5, 5, 3, 7};
arr_size = sizeof(array2)/sizeof(array2[0]);
// Printing the result of the 'test' function for 'array2'
printf("\n%d",test(array2, arr_size, 3));
// Declaration and initialization of an integer array 'array3'
int array3[] = {3, 7, 5, 5, 3, 7, 5 };
arr_size = sizeof(array3)/sizeof(array3[0]);
// Printing the result of the 'test' function for 'array3'
printf("\n%d",test(array3, arr_size, 3));
}
// Definition of the 'test' function
int test(int numbers[], int arr_size, int len)
{
// Looping through the first 'len' elements
for (int i = 0; i < len; i++)
{
// Checking if corresponding elements from the end match
if (numbers[i] != numbers[arr_size - len + i])
{
return 0; // Return 0 if there's a mismatch
}
}
return 1; // Return 1 if all elements match
}
Sample Output:
1 0 1
Pictorial Presentation:
Flowchart:
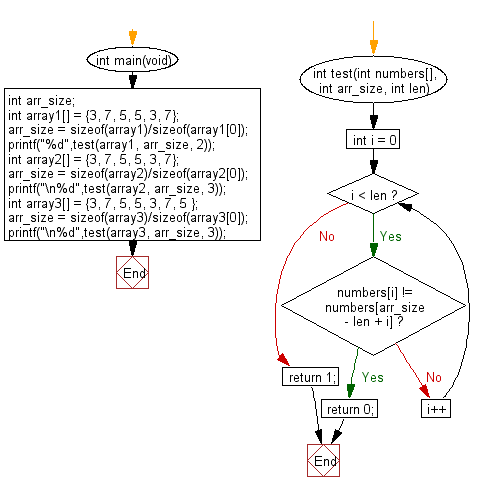
C Programming Code Editor:
Previous: Write a C program to check a given array of integers and return true if every 5 that appears in the given array is next to another 5.
Next: Write a C program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-66.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics