C Exercises: Shift an element in left direction and return a new array
68. Left Shift Array Elements
Write a C program to shift an element in the left direction and return a newly created array.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'a1'
int a1[] = {10, 20, 30, 40};
arr_size = sizeof(a1)/sizeof(a1[0]);
// Printing the elements of the original array 'a1'
printf("Elements in original array are: ");
print_array(a1, arr_size);
// Declaration and initialization of an integer array 'result'
int result[arr_size];
// Loop to generate the elements of the new array 'result'
for (int i = 0; i < arr_size; i++)
{
result[i] = a1[(i + 1) % arr_size];
}
// Printing the elements of the new array 'result'
printf("\nElements in new array are: ");
print_array(result, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
// Loop to print the elements of the array
for( i = 0; i < size - 1; i++)
{
printf("%d, ", parray[i]);
}
// Printing the last element
printf("%d ", parray[i]);
printf("\n");
}
Sample Output:
Elements in original array are: 10, 20, 30, 40 Elements in new array are: 20, 30, 40, 10
Pictorial Presentation:
Flowchart:
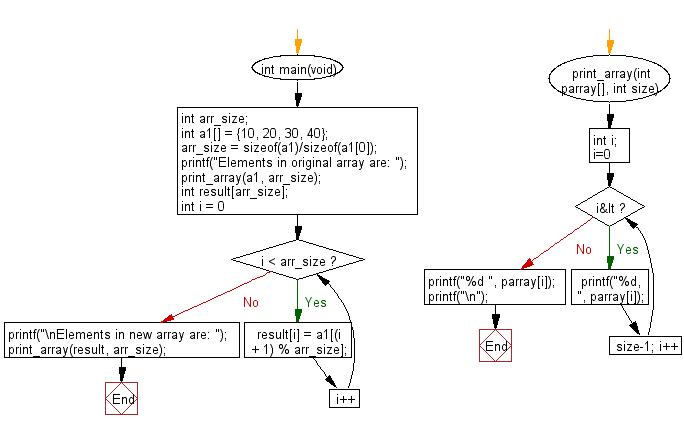
For more Practice: Solve these Related Problems:
- Write a C program to shift array elements to the right and return a new array.
- Write a C program to cyclically rotate an array by two positions to the left.
- Write a C program to shift all elements of an array left by one and insert a zero at the end.
- Write a C program to shift the elements of an array left by a user-defined number of positions.
C Programming Code Editor:
Previous: Write a C program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Next: Write a C program to create a new array taking the elements before the element value 5 from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.