C Exercises: Create a new array taking the elements after the element value 5 from a given array of integers
70. New Array After Element 5
Write a C program to create a array taking the elements after the element value 5 from a given array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'a1'
int a1[] = {1, 2, 3, 5, 7, 9, 11};
arr_size = sizeof(a1)/sizeof(a1[0]);
// Printing the elements of the original array 'a1'
printf("Elements in original array are: ");
print_array(a1, arr_size);
// Declaration of 'size' variable and calculation of the number of elements after the first 5
int size = 0;
int len = arr_size;
int i = len - 1;
// Loop to find the last occurrence of 5 in 'a1'
while (i >= 0 && a1[i] != 5) i--;
i++;
// Calculate the number of elements after the last 5
size = len - i;
// Declaration of 'post_ele_5' array with the calculated 'size'
int post_ele_5[size];
// Loop to copy elements from 'a1' after the last 5 to 'post_ele_5'
for (int j = 0; j < size; j++)
{
post_ele_5[j] = a1[i + j];
}
// Updating 'arr_size' to reflect the size of the 'post_ele_5' array
arr_size = sizeof(post_ele_5)/sizeof(post_ele_5[0]);
// Printing the elements of the new array 'post_ele_5'
printf("\nElements in new array are: ");
print_array(post_ele_5, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
// Loop to print the elements of the array
for( i = 0; i < size - 1; i++)
{
printf("%d, ", parray[i]);
}
// Printing the last element
printf("%d ", parray[i]);
printf("\n");
}
Sample Output:
Elements in original array are: 1, 2, 3, 5, 7, 9, 11 Elements in new array are: 7, 9, 11
Pictorial Presentation:
Flowchart:
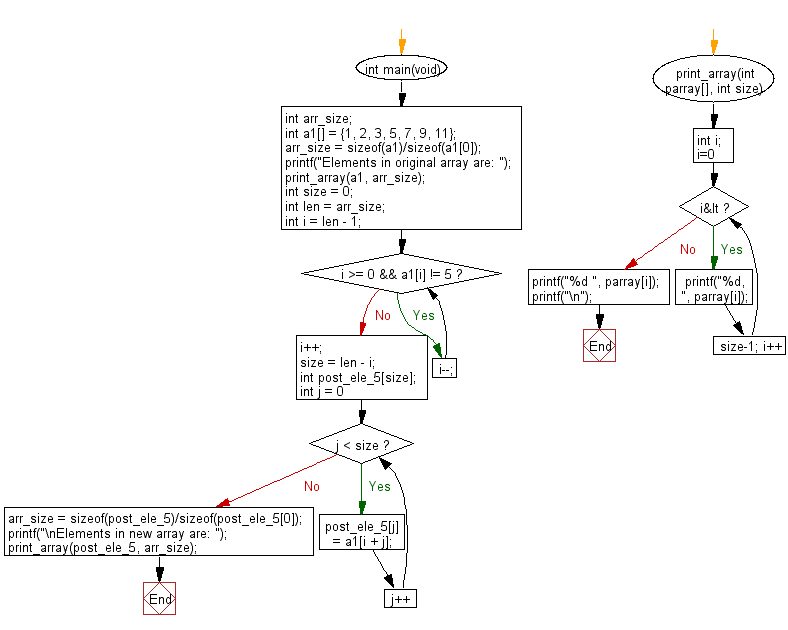
For more Practice: Solve these Related Problems:
- Write a C program to create a subarray with all elements after the first occurrence of a specific number.
- Write a C program to extract elements following a given value from an array and store them in a new array.
- Write a C program to generate a new array of elements that appear after the last occurrence of a specified number.
- Write a C program to create an array from elements occurring after a user-defined marker in an array.
C Programming Code Editor:
Previous: Write a C program to create a new array taking the elements before the element value 5 from a given array of integers.
Next: Write a C program to create a new array from a given array of integers shifting all zeros to left direction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.