C Exercises: Create a new array after replacing all the values 5 with 0 shifting all zeros to right direction
C-programming basic algorithm: Exercise-72 with Solution
Write a C program to create an array after replacing all the values 5 with 0 and shifting all zeros to the right.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
int arr_size;
// Declaration and initialization of an integer array 'a1'
int a1[] = {1, 2, 0, 3, 5, 7, 0, 9, 11, 5};
arr_size = sizeof(a1)/sizeof(a1[0]);
// Printing the elements of the original array 'a1'
printf("Elements in original array are: ");
print_array(a1, arr_size);
// Declaration of 'size' and 'index' variables
int size = arr_size, index = 0;
// Declaration of integer array 'result'
int result[size];
// Loop to iterate through the elements of 'a1'
for (int i = 0; i < size; i++)
{
// If the current element is not equal to 5, copy it to 'result' and increment 'index'
if (a1[i] != 5)
{
result[index++] = a1[i];
}
}
// Update 'arr_size' to reflect the size of the modified 'result' array
arr_size = sizeof(result)/sizeof(result[0]);
// Printing the elements of the modified array 'result'
printf("\nElements in new array are: ");
print_array(result, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
// Loop to print the elements of the array
for( i = 0; i < size - 1; i++)
{
printf("%d, ", parray[i]);
}
// Printing the last element
printf("%d ", parray[i]);
printf("\n");
}
Sample Output:
Elements in original array are: 1, 2, 0, 3, 5, 7, 0, 9, 11, 5 Elements in new array are: 1, 2, 0, 3, 7, 0, 9, 11, 0, 0
Pictorial Presentation:
Flowchart:
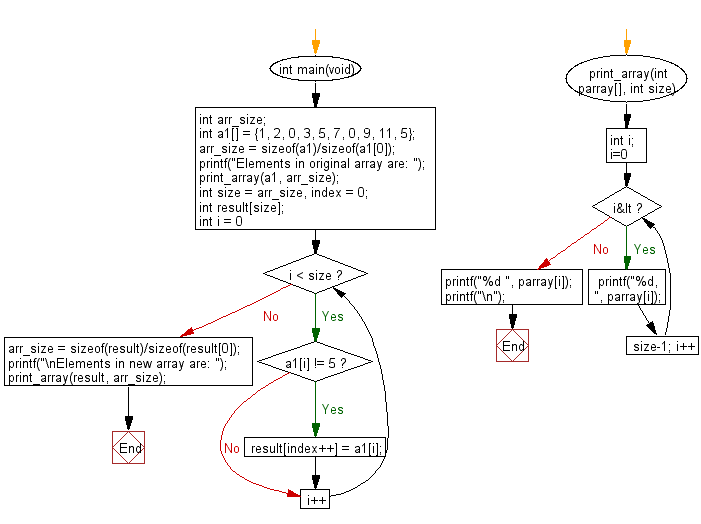
C Programming Code Editor:
Previous: Write a C program to create a new array from a given array of integers shifting all zeros to left direction.
Next: Write a C program to create new array from a given array of integers shifting all even numbers before all odd numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics