C Exercises: Check if the value of each element is equal or greater than the value of previous element of a given array of integers
C-programming basic algorithm: Exercise-74 with Solution
Write a C program to check if the value of each element is equal or greater than the value of the previous element of a given array of integers.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
int array1[] = {5, 5, 1, 5, 5};
arr_size = sizeof(array1)/sizeof(array1[0]);
printf("%d", test(array1, arr_size));
int array2[] = {1, 2, 3, 4};
arr_size = sizeof(array2)/sizeof(array2[0]);
printf("\n%d", test(array2, arr_size));
int array3[] = {3, 3, 5, 5, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
printf("\n%d", test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Loop to iterate through the array
for (int i = 0; i < arr_size - 1; i++)
{
// Check if the next element is less than the current element
if (nums[i + 1] < nums[i])
return 0; // If true, the array is not in non-decreasing order
}
return 1; // If the loop completes without returning 0, the array is in non-decreasing order
}
Sample Output:
0 1 1
Pictorial Presentation:
Flowchart:
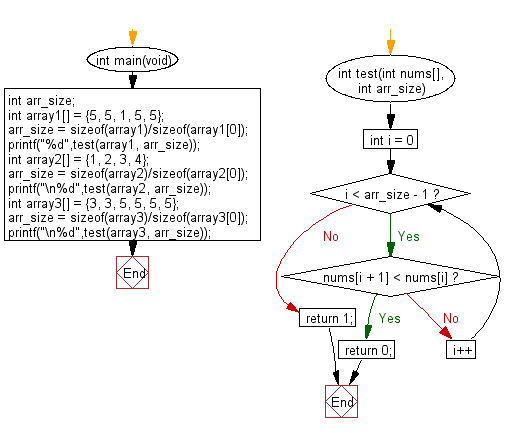
C Programming Code Editor:
Previous: Write a C program to create new array from a given array of integers shifting all even numbers before all odd numbers.
Next: Write a C program to check a given array (length will be atleast 2) of integers and return true if there are two values 15, 15 next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-74.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics