C Exercises: Check a given array of integers and return true if there are two values 15, 15 next to each other
75. Check for Adjacent 15's in Array
Write a C program to check a given array (length will be at least 2) of integers and return true if there are two values 15, 15 next to each other.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
int arr_size;
// Initialize array1 and calculate its size
int array1[] = {5, 5, 1, 15, 15};
arr_size = sizeof(array1)/sizeof(array1[0]);
// Print the result of calling 'test' with array1
printf("%d", test(array1, arr_size));
// Similar steps for array2 and array3
int array2[] = {15, 2, 3, 4, 15};
arr_size = sizeof(array2)/sizeof(array2[0]);
printf("\n%d", test(array2, arr_size));
int array3[] = {3, 3, 15, 15, 5, 5};
arr_size = sizeof(array3)/sizeof(array3[0]);
printf("\n%d", test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Loop to iterate through the array
for (int i = 0; i < arr_size - 1; i++)
{
// Check if the current and next element are both 15
if (nums[i + 1] == nums[i] && nums[i] == 15)
return 1; // If true, condition is met, so return 1
}
return 0; // If loop completes without finding the condition, return 0
}
Sample Output:
1 0 1
Pictorial Presentation:
Flowchart:
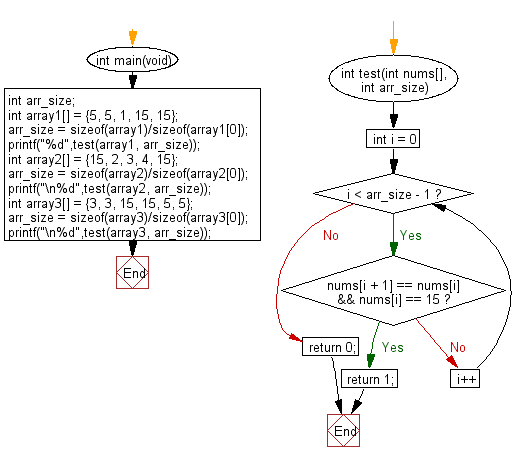
For more Practice: Solve these Related Problems:
- Write a C program to verify if an array contains adjacent pairs of the number 20.
- Write a C program to check if an array has any consecutive identical pairs of a given number.
- Write a C program to determine if two adjacent numbers in an array sum up to 30.
- Write a C program to check if an array contains at least one instance of two consecutive numbers that are both multiples of 5.
C Programming Code Editor:
Previous: Write a C program to create new array from a given array of integers shifting all even numbers before all odd numbers.
Next: C Basic Declarations and Expressions Exercises Home
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.