Calculate product of two integers
Calculate product of two integers
Write a C program that accepts two integers from the user and calculates the product of the two integers.
Pictorial Presentation:
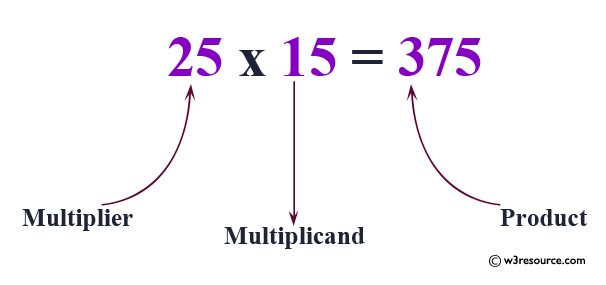
C Code:
#include <stdio.h>
int main()
{
int x, y, result; // Declare variables for two integers and their product
// Prompt user for input and store in 'x'
printf("\nInput the first integer: ");
scanf("%d", &x);
// Prompt user for input and store in 'y'
printf("\nInput the second integer: ");
scanf("%d", &y);
result = x * y; // Calculate the product of 'x' and 'y'
// Print the product
printf("Product of the above two integers = %d\n", result);
}
Sample Output:
Input the first integer: 25 Input the second integer: 15 Product of the above two integers = 375
Flowchart:
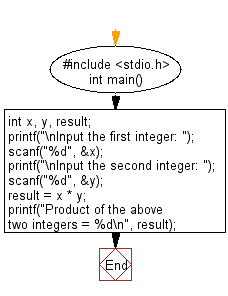
For more Practice: Solve these Related Problems:
- Write a C program to calculate the product of three integers provided by the user.
- Write a C program to compute the product of two integers without using the multiplication operator, relying on repeated addition.
- Write a C program to calculate both the product and the sum of two integers and display both results.
- Write a C program to recursively compute the product of two integers using a custom recursive function.
C Programming Code Editor:
Previous: Write a C program that accepts two integers from the user and calculate the sum of the two integers.
Next: Write a C program that accepts two item’s weight (floating points' values ) and number of purchase (floating points' values) and calculate the average value of the items.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.