Calculate average weight for purchases
Calculate average weight for purchases
Write a C program that accepts two item's weight and number of purchases (floating point values) and calculates their average value.
C Code:
#include <stdio.h>
int main()
{
double wi1, ci1, wi2, ci2, result; // Declare variables for weights and counts
// Prompt user for weight and count of item 1
printf("Weight - Item1: ");
scanf("%lf", &wi1);
// Prompt user for count of item 1
printf("No. of item1: ");
scanf("%lf", &ci1);
// Prompt user for weight and count of item 2
printf("Weight - Item2: ");
scanf("%lf", &wi2);
// Prompt user for count of item 2
printf("No. of item2: ");
scanf("%lf", &ci2);
// Calculate average value
result = ((wi1 * ci1) + (wi2 * ci2)) / (ci1 + ci2);
// Print the average value
printf("Average Value = %f\n", result);
return 0;
}
Sample Output:
Weight - Item1: 15 No. of item1: 5 Weight - Item2: 25 No. of item2: 4 Average Value = 19.444444
Flowchart:
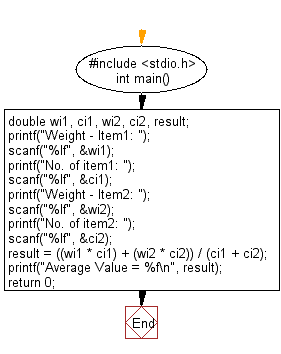
For more Practice: Solve these Related Problems:
- Write a C program to compute the weighted average of two items given their weights and corresponding purchase quantities.
- Write a C program to calculate the average weight and variance of purchase items from multiple inputs.
- Write a C program to dynamically read weights and quantities for an unknown number of items, then calculate the overall average.
- Write a C program to calculate the average price per unit weight when given total cost and total weight of purchases.
C Programming Code Editor:
Previous: Write a C program that accepts two integers from the user and calculate the product of the two integers.
Next: Write a C program that accepts an employee's ID, total worked hours of a month and the amount he received per hour. Print the employee's ID and salary (with two decimal places) of a particular month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.