C Exercises: New item price and increased price according to the item price table
Revise an item's price based on a price table
Write a C program that reads the item's price and creates a revised price for the item, based on the item price table.
Item Price | Increase Rate |
---|---|
100 - 400.00 | 14% |
400.01 - 800.00 | 11% |
800.01 - 1200.00 | 9% |
1200.01 - 2000.00 | 6% |
Above 2000.00 | 3% |
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
// Declare variables for item price and increased price
float price, increased_price;
// Prompt the user to input the item price
printf("Input the item price:");
// Read the input value for item price
scanf("%f", &price);
// Check the range of the item price and calculate increased price and percentage accordingly
if (price >= 100 && price <= 400){
// Calculate increased price and update item price
increased_price = price * 0.14;
price = price + increased_price;
// Print the new item price, increased price, and increase percentage
printf("New Item price: %.2f\n", price);
printf("Increased price: %.2f\n", increased_price);
printf("Increase Percentage: 14%%\n");
}
else{
if (price > 400 && price <= 800){
increased_price = price * 0.11;
price = price + increased_price;
printf("New Item price: %.2f\n", price);
printf("Increased price: %.2f\n", increased_price);
printf("Increase Percentage: 11%%\n");
}
else{
if (price > 800 && price <= 1200){
increased_price = price * 0.09;
price = price + increased_price;
printf("New Item price: %.2f\n", price);
printf("Increased price: %.2f\n", increased_price);
printf("Increase Percentage: 9%%\n");
}
else{
if (price > 1200 && price <= 2000){
increased_price = price * 0.06;
price = price + increased_price;
printf("New Item price: %.2f\n", price);
printf("Increased price: %.2f\n", increased_price);
printf("Increase Percentage: 6%%\n");
}
else{
increased_price = price * 0.03;
price = price + increased_price;
printf("New Item price: %.2f\n", price);
printf("Increased price: %.2f\n", increased_price);
printf("Increase Percentage: 3%%\n");
}
}
}
}
}
Sample Output:
Input the item price:525 New Item price: 582.75 Increased price: 57.75 Increase Percentage: 11%
Flowchart:
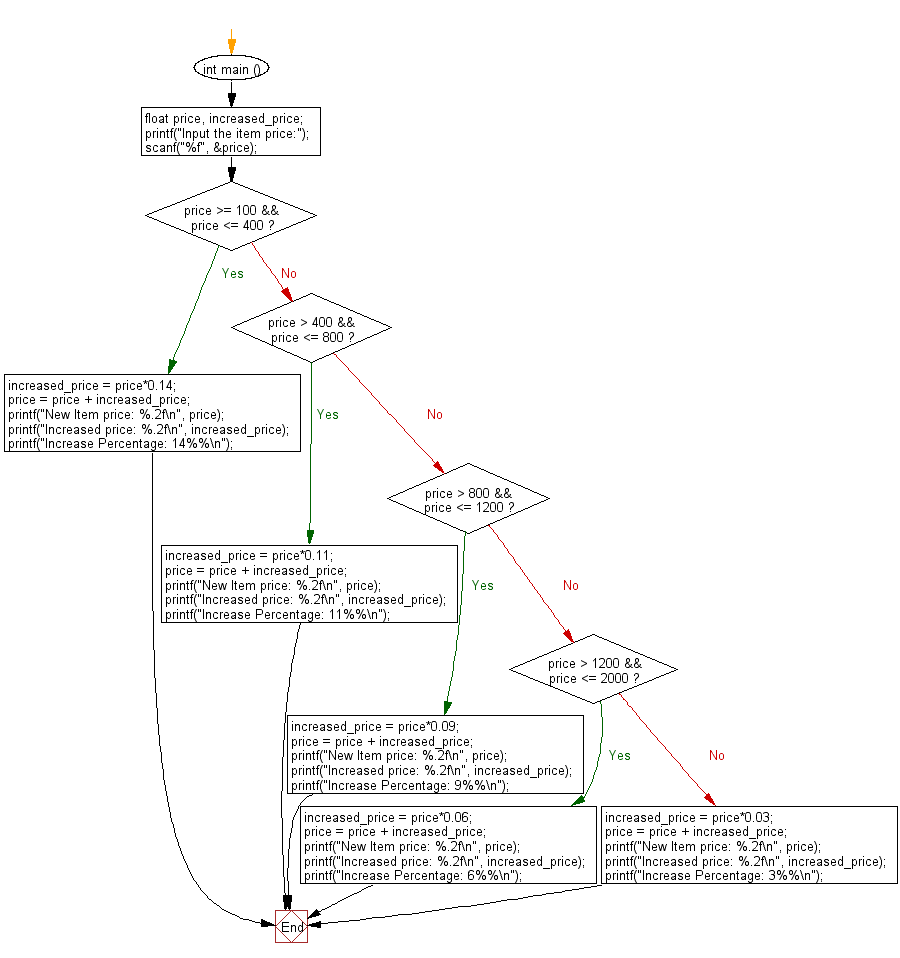
For more Practice: Solve these Related Problems:
- Write a C program to adjust an item’s price using tiered percentage increases from a price table.
- Write a C program to compute a revised price based on a discount/markup table using switch-case logic.
- Write a C program that calculates the new price and percentage increase using function pointers for different price ranges.
- Write a C program to determine the revised price by comparing the item’s price against multiple thresholds and applying complex conditions.
C programming Code Editor:
Previous: Write a C program that takes two integers and test whether they are multiplies are not.
Next: Write a C program that accepts seven floating point numbers and count the number of positive and negative numbers. Also print the average of all positive and negative values with two digit after the decimal number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.