C Exercises: Count and compute positive, negative numbers and average with two digits after the decimal number
Count positive/negative numbers and calculate their averages
Write a C program that accepts seven floating point numbers and counts the number of positive and negative numbers. Print the average of all positive and negative values with two digits after the decimal number.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
float x, p_avg = 0, n_avg = 0, temp_p = 0, temp_n = 0;
int i, p_ctr = 0, n_ctr = 0;
// Prompt the user to input 7 numbers
printf("Input 7 numbers(int/float):\n");
// Loop through 7 times to read the numbers
for (i = 0; i < 7; i++){
// Read a number
scanf("%f", &x);
// Check if the number is positive
if (x > 0){
p_ctr++; // Increment positive counter
temp_p += x; // Sum positive numbers
}
// Check if the number is negative
if (x < 0){
n_ctr++; // Increment negative counter
temp_n += x; // Sum negative numbers
}
}
// Calculate the average of positive numbers
p_avg = temp_p/p_ctr;
// Calculate the average of negative numbers
n_avg = temp_n/n_ctr;
// Check if there were positive numbers
if (p_ctr > 0){
printf("\n%d Number of positive numbers: ", p_ctr);
printf("Average %.2f\n", p_avg);
}
// Check if there were negative numbers
if (n_ctr > 0){
printf("\n%d Number of negative numbers: ", n_ctr);
printf("Average %.2f\n", n_avg);
}
}
Sample Output:
Input 7 numbers(int/float): 25 35.75 15 -3.5 40 35 16 6 Number of positive numbers: Average 27.79 1 Number of negative numbers: Average -3.50
Flowchart:
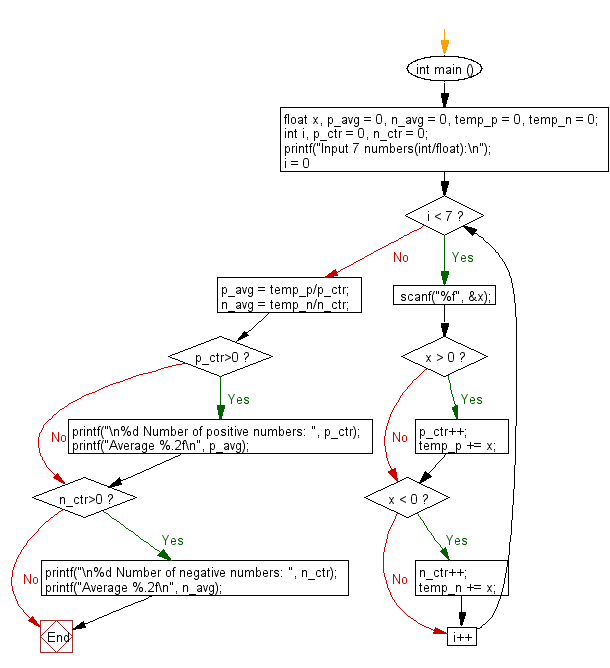
For more Practice: Solve these Related Problems:
- Write a C program to read seven floats, separate them into positive and negative groups, and compute each group’s average using loops.
- Write a C program to count positive and negative numbers and compute their averages using arrays and pointer arithmetic.
- Write a C program to calculate the averages of positive and negative values from a set of inputs with error-checking for non-numeric entries.
- Write a C program to segregate numbers into positive and negative buckets, compute their averages, and display results with formatted output.
C programming Code Editor:
Previous: Write a C program that read the item's price and create new item price and increased price of that item according to the item price table.
Next: Write a C program that accepts 7 integer values and count the even, odd, positive and negative values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.