C Exercises: Accept an integer and print next ten consecutive odd and even numbers
Print next 10 odd and even numbers after a given integer
Write a C program that prints ten consecutive odd and even numbers after accepting an integer.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int a, i, ctr = 0;
// Prompt the user to input an integer number
printf("Input an integer number:\n");
scanf("%d", &a);
// Print the next 10 consecutive odd numbers
printf("\nNext 10 consecutive odd numbers:\n");
for (i = a + 1; ctr < 10; i++){
if (i % 2 != 0){
printf("%d, ", i);
ctr++;
}
}
// Reset counter for even numbers
ctr = 0;
// Print the next 10 consecutive even numbers
printf("\n\nNext 10 consecutive even numbers:\n");
for (i = a + 1; ctr < 10; i++){
if (i % 2 == 0){
printf("%d, ", i);
ctr++;
}
}
}
Sample Output:
Input an integer number: 15 Next 10 consecutive odd numbers: 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, Next 10 consecutive even numbers: 26, 28, 30, 32, 34, 36, 38, 40, 42, 44,
Flowchart:
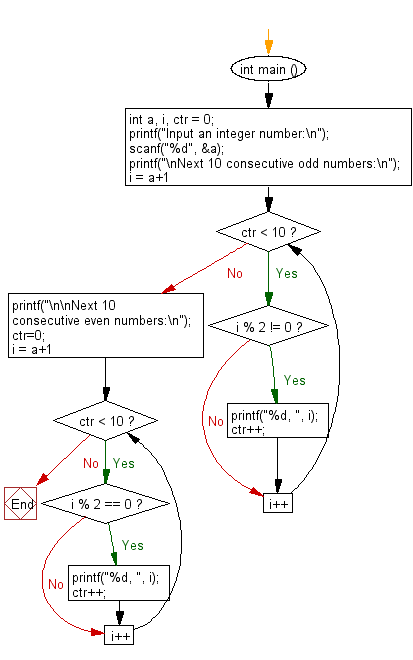
For more Practice: Solve these Related Problems:
- Write a C program to generate the next ten odd and even numbers using modular arithmetic and loops.
- Write a C program to compute and display two separate sequences: one for the next ten odd and one for the next ten even numbers.
- Write a C program that uses recursion to print the next ten odd and even numbers starting from a given integer.
- Write a C program to accept an integer and then use two functions to print the subsequent odd and even sequences concurrently.
C programming Code Editor:
Previous: Write a C program that accepts 7 integer values and count the even, odd, positive and negative values.
Next: Write a C program that reads two integer values and calculate the sum of all odd and values between them.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.