C Exercises: Create and print a specified sequence
Generate and print a sequence
Write a C program to create and print the sequence of the following example.
a=1 b=100
a=6 b=90
a=11 b=80
a=16 b=70
a=21 b=60
a=26 b=50
a=31 b=40
a=36 b=30
a=41 b=20
a=46 b=10
a=51 b=0
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
int a, b;
// Loop to execute statements with changing values of 'a' and 'b'
for (a = 1, b = 100; b >= 0; a += 5, b -= 10)
printf("a=%d \t b=%d\n", a, b);
}
Sample Output:
a=1 b=100 a=6 b=90 a=11 b=80 a=16 b=70 a=21 b=60 a=26 b=50 a=31 b=40 a=36 b=30 a=41 b=20 a=46 b=10 a=51 b=0
Flowchart:
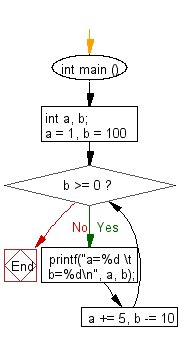
For more Practice: Solve these Related Problems:
- Write a C program to generate a sequence by incrementing one variable and decrementing another simultaneously.
- Write a C program to print two parallel sequences that converge toward each other using loops.
- Write a C program to create a sequence where one series increases by a fixed step and the other decreases, then print them side by side.
- Write a C program to generate a custom sequence with dual variables updating in opposite directions and display the results in a table.
C programming Code Editor:
Previous: Write a C program that reads seven integer values from the user and find the highest value and it’s position.
Next: Write a C program that accepts two integers values and calculate the sum of all even values between them, exclusive input values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.