C Exercises: Find Ascending or Descending order from a pair of numbers
Check if two integers are in ascending or descending order
Write a C program that accepts a pair of numbers from the user and prints "Ascending order" if the two numbers are in ascending order, otherwise prints, "Descending order".
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int a, b;
// Prompt user for input
printf("Input two pairs values (integer values):\n");
// Read two integer values from user and store them in 'a' and 'b'
scanf("%d %d", &a, &b);
// Check if 'a' is not equal to 'b'
if (a != b) {
// Check if 'b' is greater than 'a'
if (b > a) {
printf("Ascending order\n"); // Print message for ascending order
}
else {
printf("Descending order\n"); // Print message for descending order
}
}
return 0; // End of program
}
Sample Output:
Input two pairs values (integer values): 12 35 Ascending order
Sample Output:
Input two pairs values (integer values): 65 25 Descending order
Flowchart:
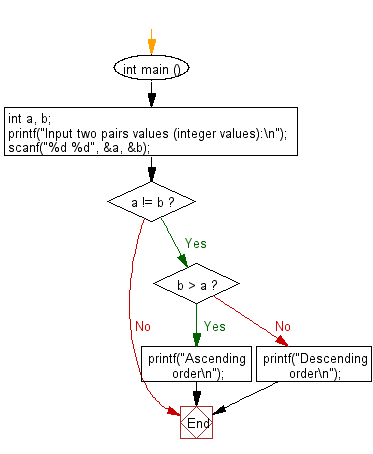
For more Practice: Solve these Related Problems:
- Write a C program to compare two integers and print "Ascending order" or "Descending order" using if-else statements.
- Write a C program to read a pair of numbers and determine their order using a ternary operator.
- Write a C program to accept two integers and use a function to return the order status as a string.
- Write a C program to determine order by subtracting the two numbers and checking the sign of the result.
C programming Code Editor:
Previous: Write a C program that accepts a pair of numbers from the user and print the sequence from the lowest to highest number. Also, print the average value of the sequence.
Next: Write a C program that read two integers and dividing the first number by second, print the result of this division with two digits after the decimal point and print "Division not possible..!" if the division is not possible.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.