C Exercises: Read two integers and dividing the first number by second
Divide two integers and print result or "Division not possible"
Write a C program that reads two integers and divides the first number by second, print the result of this division with two digits after the decimal point and prints "Division not possible..!" if the division is not possible.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int n, x, y, i;
float result = 0;
// Prompt user for input
printf("Input two integer values:\n");
// Read two integer values from user and store them in 'x' and 'y'
scanf("%d %d", &x, &y);
// Check if 'y' is zero (division by zero case)
if (y == 0) {
printf("Division not possible..!\n"); // Print an error message
}
else {
// Calculate the result of division as a float value
result = (x * 1.0) / (y);
// Print the result with two decimal places
printf("Result: %.2f\n", result);
}
return 0; // End of program
}
Sample Output:
Input two integer values: 75 5 Result: 15.00
Flowchart:
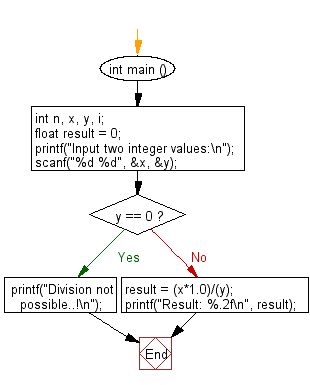
For more Practice: Solve these Related Problems:
- Write a C program to perform division of two integers and display the result with fixed decimal precision, handling division by zero.
- Write a C program to divide two numbers and use error-checking to print a custom message if the divisor is zero.
- Write a C program to implement a division function that returns a flag for division not possible along with the result.
- Write a C program to perform integer division and output both the quotient and remainder, with error handling for zero divisor.
C programming Code Editor:
Previous: Write a C program that accepts a pair of numbers from the user and prints "Ascending order" if the two numbers are in ascending order, otherwise prints, "Descending order".
Next: Write a C program that reads five subject marks (0-100) of a student and calculate the average of these marks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.