C Exercises: Read five subject marks (0-100) of a student and calculate the average
Calculate the average of marks from 5 subjects
Write a C program that reads five subject marks (0-100) of a student and calculates the average of these marks.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
float marks, tot_marks = 0;
int i = 0, subject = 0;
// Prompt user for input
printf("Input five subject marks(0-100):\n");
// Loop to read marks for five subjects
while (subject != 5) {
// Read a float value 'marks' from user
scanf("%f", &marks);
// Check if 'marks' is within valid range (0-100)
if (marks < 0 || marks > 100) {
printf("Not a valid marks\n"); // Print an error message
}
else {
tot_marks += marks; // Accumulate valid marks
subject++; // Increment subject count
}
}
// Calculate and print the average marks
printf("Average marks = %.2f\n", tot_marks/5);
return 0; // End of program
}
Sample Output:
Input five subject marks(0-100): 75 84 56 98 68 Average marks = 76.20
Flowchart:
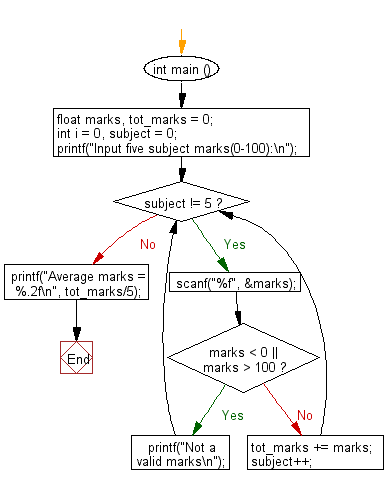
For more Practice: Solve these Related Problems:
- Write a C program to read five subject marks, validate each input, and compute the average with two decimal precision.
- Write a C program to calculate the average marks using an array and a loop, ensuring values are between 0 and 100.
- Write a C program to implement a function that computes the average of five marks and prints a grade based on the result.
- Write a C program to compute the average of marks, and then display a message if the student passes or fails based on a threshold.
C programming Code Editor:
Previous: Write a C program that read two integers and dividing the first number by second, print the result of this division with two digits after the decimal point and print "Division not possible..!" if the division is not possible.
Next: Write a C program to calculate the sum all numbers between two given numbers (inclusive) not divisible by 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.