C Exercises: Sum all numbers between two given numbers (inclusive) not divisible by seven
C Basic Declarations and Expressions: Exercise-119 with Solution
Write a C program to calculate the sum of all numbers between two given numbers (inclusive) not divisible by 7.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int a, b, sum_nums = 0, i, temp = 0;
// Prompt user for input
printf("Input two numbers(integer):\n");
// Read two integer values from user and store them in 'a' and 'b'
scanf("%d %d", &a, &b);
// Swap 'a' and 'b' if 'b' is smaller than 'a'
if (b < a) {
temp = a;
a = b;
b = temp;
}
// Loop through the range from 'a' to 'b' (inclusive)
for (i = a; i <= b; i++) {
// Check if 'i' is not divisible by 7
if (i % 7 != 0) {
sum_nums += i; // Accumulate numbers not divisible by 7
}
}
// Print the sum of numbers not divisible by 7
printf("Sum of all numbers between said numbers (inclusive) not divisible by 7:\n");
printf("%d\n", sum_nums);
return 0; // End of program
}
Sample Output:
Input two numbers(integer): 25 5 Sum of all numbers between said numbers (inclusive) not divisible by 7: 273
Sample Output:
Input two numbers(integer): 6 36 Sum of all numbers between said numbers (inclusive) not divisible by 7: 546
Flowchart:
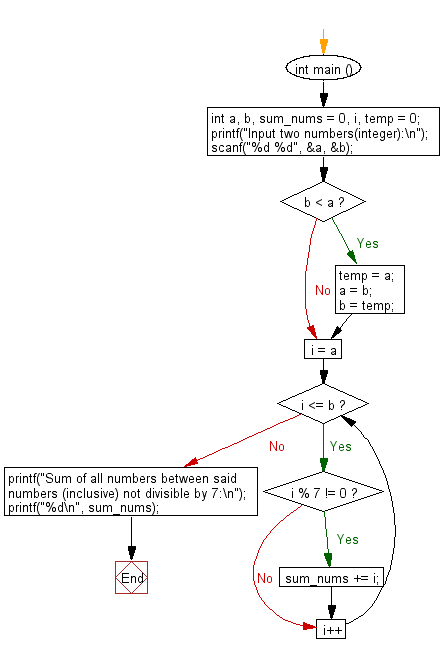
C programming Code Editor:
Previous: Write a C program that reads five subject marks (0-100) of a student and calculate the average of these marks.
Next: Write a C program to print a sequence from 1 to a given (integer) number, insert a comma between these numbers, there will be no comma after the last character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-declarations-and-expressions/c-programming-basic-exercises-119.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics