C Exercises: Insert a comma between two numbers, no comma after the last character
Print sequence from 1 to nnn separated by commas
Write a C program to print a sequence from 1 to a given (integer) number, inserting a comma between these numbers. There will be no comma after the last character.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int n, i;
// Prompt user for input
printf("\nInput a number(integer):\n");
// Read an integer value 'n' from user
scanf("%d", &n);
// Check if 'n' is positive
if (n > 0) {
printf("Sequence:\n");
// Loop to print numbers from 1 to 'n-1'
for (i = 1; i < n; i++) {
printf("%d,", i); // Print each number followed by a comma
}
printf("%d\n", i); // Print the last number without a comma
}
}
Sample Output:
Input a number(integer): 25 Sequence: 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25
Flowchart:
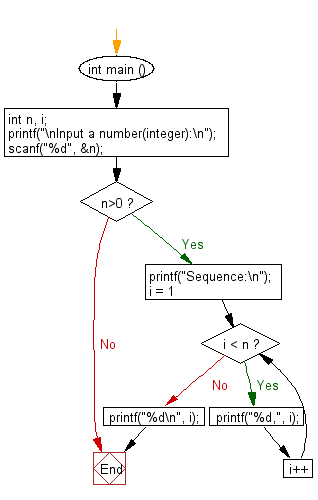
For more Practice: Solve these Related Problems:
- Write a C program to print numbers from 1 to n with commas between them, ensuring no trailing comma using loops.
- Write a C program to generate a comma-separated sequence using pointer arithmetic and string manipulation.
- Write a C program to print the sequence from 1 to n using recursion and concatenating commas appropriately.
- Write a C program to build the sequence in an array and then output it as a comma-separated string.
C programming Code Editor:
Previous: Write a C program to calculate the sum all numbers between two given numbers (inclusive) not divisible by 7.
Next: Write a C program that reads an integer and find all the divisors of the said integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.