C Exercises: Read an integer and compute all its divisors
Find and print all divisors of an integer
Write a C program that reads an integer and finds all the divisors of the said integer.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int i, n;
// Prompt user for input
printf("Input a number (integer value):\n");
// Read an integer value 'n' from user
scanf("%d", &n);
// Print a message indicating what the program will do
printf("\nAll positive divisors of %d \n",n);
// Loop through numbers from 1 to 'n'
for (i = 1; i <= n; i++) {
// Check if 'i' is a divisor of 'n'
if (n % i == 0) {
printf("%d\n", i); // Print 'i' if it is a divisor of 'n'
}
}
return 0; // End of program
}
Sample Output:
Input a number (integer value): 35 All positive divisors of 35 1 5 7 35
Flowchart:
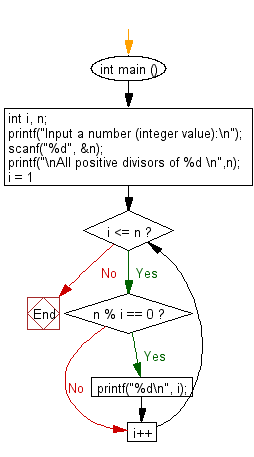
For more Practice: Solve these Related Problems:
- Write a C program to find all positive divisors of an integer using a loop with conditional modulus checks.
- Write a C program to store all divisors of a number in an array and then print them in sorted order.
- Write a C program to recursively determine and print all divisors of a given integer.
- Write a C program to optimize divisor search by iterating only up to the square root of the number and printing corresponding pairs.
C programming Code Editor:
Previous: Write a C program to print a sequence from 1 to a given (integer) number, insert a comma between these numbers, there will be no comma after the last character.
Next: Write a C program that reads two integers m, n and compute the sum of n even numbers starting from m.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.