C Exercises: Sum of n even numbers starting from m
C Basic Declarations and Expressions: Exercise-122 with Solution
Compute sum of nnn even numbers starting from mmm
Write a C program that reads two integers m, n and computes the sum of n even numbers starting from m.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int m, n, i, j, k, sum_even_nums = 0;
// Prompt user for input
printf("\nInput two integers (m, n):\n");
// Read two integer values 'm' and 'n' from user
scanf("%d %d", &m, &n);
// Print a message indicating what the program will do
printf("\nSum of %d even numbers starting from %d: ",n,m);
// Loop to find and sum 'n' even numbers starting from 'm'
for (k = 0, j = m; k < n; j++) {
// Check if 'j' is even
if (j % 2 == 0) {
sum_even_nums += j; // Accumulate even numbers
k++; // Increment the count of even numbers found
}
}
// Print the sum of even numbers
printf("\n%d", sum_even_nums);
return 0; // End of program
}
Sample Output:
Input two integes (m, n): 20 60 Sum of 60 even numbers starting from 20: 4740
Flowchart:
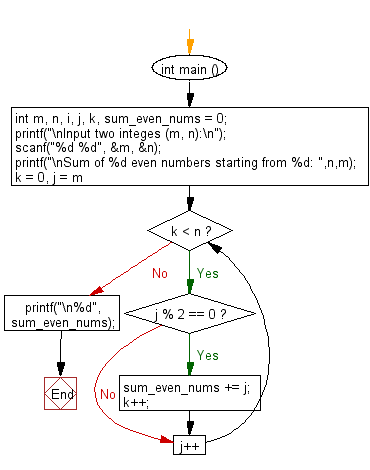
C programming Code Editor:
Previous: Write a C program that reads an integer and find all the divisors of the said integer.
Next: Write a C program that reads two integers m, n and compute the sum of n odd numbers starting from m.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-declarations-and-expressions/c-programming-basic-exercises-122.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics