C Exercises: Sum of n odd numbers starting from m
Compute sum of nnn odd numbers starting from mmm
Write a C program that reads two integers m, n and computes the sum of n odd numbers starting from m.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int m, n, i, j, k, sum_odd_nums = 0;
// Prompt user for input
printf("\nInput two integers (m, n):\n");
// Read two integer values 'm' and 'n' from user
scanf("%d %d", &m, &n);
// Print a message indicating what the program will do
printf("\nSum of %d odd numbers starting from %d: ",n,m);
// Loop to find and sum 'n' odd numbers starting from 'm'
for (k = 0, j = m; k < n; j++) {
// Check if 'j' is odd
if (j % 2 != 0) {
sum_odd_nums += j; // Accumulate odd numbers
k++; // Increment the count of odd numbers found
}
}
// Print the sum of odd numbers
printf("\n%d", sum_odd_nums);
return 0; // End of program
}
Sample Output:
Input two integes (m, n): 65 5 Sum of 5 odd numbers starting from 65: 345
Flowchart:
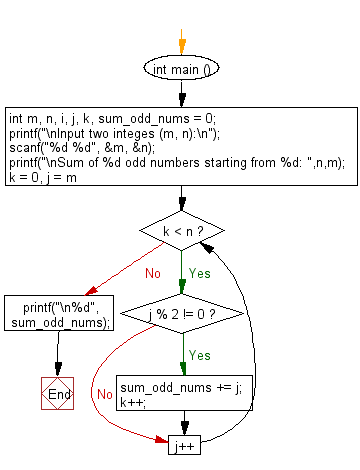
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of n consecutive odd numbers starting from m using a loop.
- Write a C program to generate the odd number sequence starting from m and compute their sum with a for-loop.
- Write a C program to accumulate the sum of n odd numbers using recursion from a starting value m.
- Write a C program to compute the sum of odd numbers using the arithmetic series formula after generating the sequence.
C programming Code Editor:
Previous: Write a C program that reads two integers m, n and compute the sum of n even numbers starting from m.
Next: Write a C program that reads an array of integers (length 7), replace every negative or null element by 1 and print the array elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.