C Exercises: Array fill, replace each subsequent position of the array by one-third value of the previous
Replace array elements with one-third of the previous
Write a C program that reads an array (length 10), and replaces the first element of the array by a given number and replaces each subsequent position of the array by one-third the value of the previous.
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
int i;
double array_nums[10];
double n;
// Prompt user for input
printf("Input a number:\n");
scanf("%lf", &n);
// Initialize the first element of the array with the input value
array_nums[0] = n;
// Loop to generate and store subsequent array elements
for (i = 1; i < 10; i++)
{
n = n / 3; // Divide the current value by 3
array_nums[i] = n; // Store the result in the array
}
// Print the array elements
printf("\nArray elements:\n");
for (i = 0; i < 10; i++)
{
printf("array_nums[%d] = %.4lf\n", i, array_nums[i]);
}
return 0; // End of program
}
Sample Output:
Input a number: 35 Array elements: array_nums[0] = 35.0000 array_nums[1] = 11.6667 array_nums[2] = 3.8889 array_nums[3] = 1.2963 array_nums[4] = 0.4321 array_nums[5] = 0.1440 array_nums[6] = 0.0480 array_nums[7] = 0.0160 array_nums[8] = 0.0053 array_nums[9] = 0.0018
Flowchart:
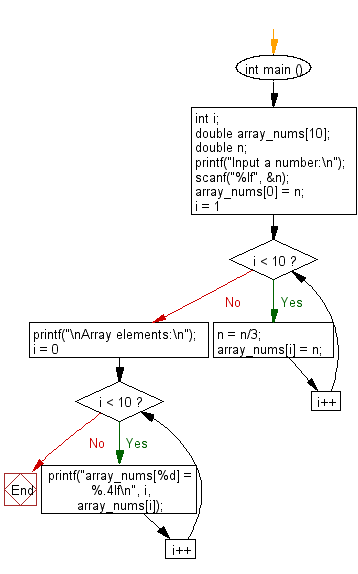
For more Practice: Solve these Related Problems:
- Write a C program to modify an array so that each element (after the first) is one-third of its predecessor using loops.
- Write a C program to implement a function that updates an array with each element equal to one-third the previous element.
- Write a C program to use pointer arithmetic to replace each element in an array with one-third of the preceding element.
- Write a C program to recursively update an array where every element becomes one-third of the previous value.
C programming Code Editor:
Previous: Write a C program that reads an array of integers (length 10), fill the array elements with number o to a (given number) n – 1 repeated times where 2 <= n <= 10.
Next: Write a C program to create an array of length n and fill the array elements with integer values. Now find the smallest value and it’s position within the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.