C Exercises: Check if a point (x, y) is within a triangle or not
C Basic Declarations and Expressions: Exercise-137 with Solution
Write a C program to check if a point (x, y) is within a triangle or not. Three points make up a triangle.
Input:
x1,y1,x2,y2,x3,y3,xp,yp separated by a single space
Sample Solution:
C Code:
#include <stdio.h>
// Function to calculate the outer product of two vectors
double check_outer_product(double X1, double Y1, double X2, double Y2) {
return X1 * Y2 - X2 * Y2;
}
int main() {
double x[3], y[3], xp, yp, cop1, cop2, cop3;
// Prompting the user to input three points to form a triangle
printf("Input three points to form a triangle:\n");
scanf("%lf %lf %lf %lf %lf %lf", &x[0], &y[0], &x[1], &y[1], &x[2], &y[2]);
// Prompting the user to input a point to check if it's inside the triangle
printf("\nInput the point to check if it is inside the triangle or not:\n");
scanf("%lf %lf", &xp, &yp);
// Calculating the outer products using the function
cop1 = check_outer_product(x[1] - x[0], y[1] - y[0], xp - x[0], yp - y[0]);
cop2 = check_outer_product(x[2] - x[1], y[2] - y[1], xp - x[1], yp - y[1]);
cop3 = check_outer_product(x[0] - x[2], y[0] - y[2], xp - x[2], yp - y[2]);
// Checking if the point is inside or outside the triangle based on outer products
if (((cop1 > 0.0) && (cop2 > 0.0) && (cop3 > 0.0)) ||
((cop1 < 0.0) && (cop2 < 0.0) && (cop3 < 0.0))) {
printf("The point is inside the triangle!");
} else {
printf("The point is outside the triangle!");
}
return 0; // End of the program
}
Sample Output:
Input three points to form a triangle: x1 y1 z1 Input the point to check it is inside the triangle or not: The point is outside the triangle!
Flowchart:
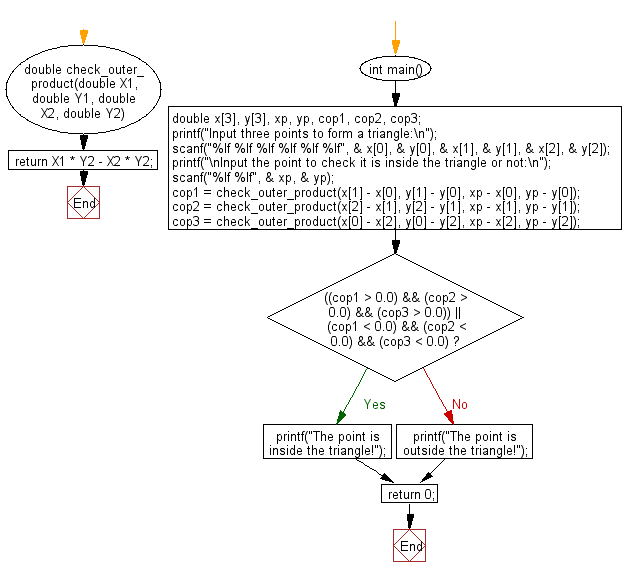
C programming Code Editor:
Previous: Write a C program to find the prime numbers which are less than or equal to a given integer.
Next: Write a C program to test whether two lines are parallel or not. The four points are P(x1, y1), Q(x2, y2), R(x3, y3) and S(x4, y4), check PQ and RS are parallel are not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics