C Exercises: Check two lines are parallel or not
Determine if two lines are parallel
Write a C program to test whether two lines are parallel or not. The four points are P(x1, y1), Q(x2, y2), R(x3, y3) and S(x4, y4), check PQ and RS are parallel are not.
Input:
−100 <= x1, y1, x2, y2, x3, y3, x4, y4 <= 100
Each value is a real number with at most 5 digits after the decimal point.
Sample Solution:
C Code:
#include <stdio.h>
main() {
// Variable declarations
double x1, x2, y1, y2, x3, y3, x4, y4;
int i, n;
// Getting user input for coordinates
printf("Input P(x1,y1):\n");
scanf("%lf %lf", &x1, &y1);
printf("\nInput P(x2,y2):\n");
scanf("%lf %lf", &x2, &y2);
printf("\nInput P(x3,y3):\n");
scanf("%lf %lf", &x3, &y3);
printf("\nInput P(x4,y4):\n");
scanf("%lf %lf", &x4, &y4);
// Checking if lines PQ and RS are parallel
if ((x1 == x2) && (x3 == x4))
printf("\nPQ and RS are parallel!\n");
else if ((x1 == x2) || (x3 == x4))
printf("\nPQ and RS are not parallel!\n");
else if (((y1 - y2) / (x1 - x2) - (y3 - y4) / (x3 - x4)) == 0.0)
printf("\nPQ and RS are parallel!\n");
else
printf("\nPQ and RS are not parallel!\n");
return (0); // End of the program
}
Sample Output:
Input P(x1,y1): 5 7 Input P(x2,y2): 3 6 Input P(x3,y3): 8 9 Input P(x4,y4): 5 6 PQ and RS are not parallel!
Flowchart:
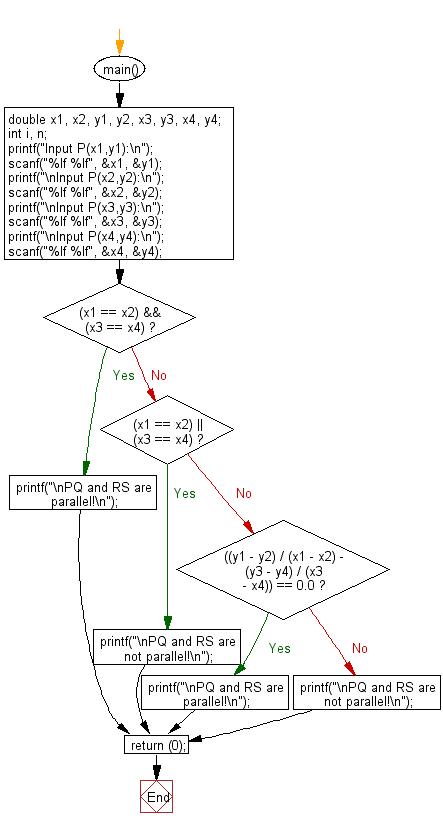
For more Practice: Solve these Related Problems:
- Write a C program to compute slopes of two lines from given endpoints and check for equality.
- Write a C program to determine if two lines are parallel by comparing the cross product of their direction vectors.
- Write a C program to use floating-point arithmetic to calculate slopes and verify parallelism with tolerance.
- Write a C program to determine parallel lines using a function that returns the slope and then compares them.
C programming Code Editor:
Previous: Write a C program to check if a point (x, y) is within a triangle or not. The triangle has formed by three points.
Next: Write a C program to find the maximum sum of a contiguous subsequence from a given sequence of numbers a1, a2, a3, ... an ( n = number of terms in the sequence).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.