C Exercises: Extract words of 3 to 6 characters length from a given sentence
Extract words of 3 to 6 characters from a sentence
Write a C program to extract words of 3 to 6 characters length from a given sentence not more than 1024 characters.
Internet search engine giant, such as Google accepts web pages around the world and classify them, creating a huge database. The search engines also analyze the search keywords entered by the user and create inquiries for database search. In both cases, complicated processing is carried out in order to realize efficient retrieval, but basics are all cutting out words from sentences.
Input:
English sentences consisting of delimiters and alphanumeric characters are given on one line.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
int main() {
// Define an array to store input text
char input_text[1536];
// Initialize a counter variable
int ctr = 0;
// Define a pointer variable 'temp'
char * temp;
// Prompt user for input
printf("English sentences consisting of delimiters and alphanumeric characters on one line:\n");
// Read a line of text from the user
fgets(input_text, sizeof(input_text), stdin);
// Print message indicating the purpose of the operation
printf("\nExtract words of 3 to 6 characters length from the said sentence:\n");
// Tokenize the input text using delimiters (space, period, comma, newline)
for (temp = strtok(input_text, " .,\n"); temp != NULL; temp = strtok(NULL, " .,\n")) {
const int len = strlen(temp);
// Check if the length of the word is between 3 and 6 characters
if (3 <= len && len <= 6) {
// If not the first word, print a space before the word
if (ctr++) putchar(' ');
// Print the word
fputs(temp, stdout);
}
}
// Print a newline character after the extracted words
puts("");
return (0);
}
Sample Output:
English sentences consisting of delimiters and alphanumeric characters on one line: w3resource.com Extract words of 3 to 6 characters length from the said sentence: com
Flowchart:
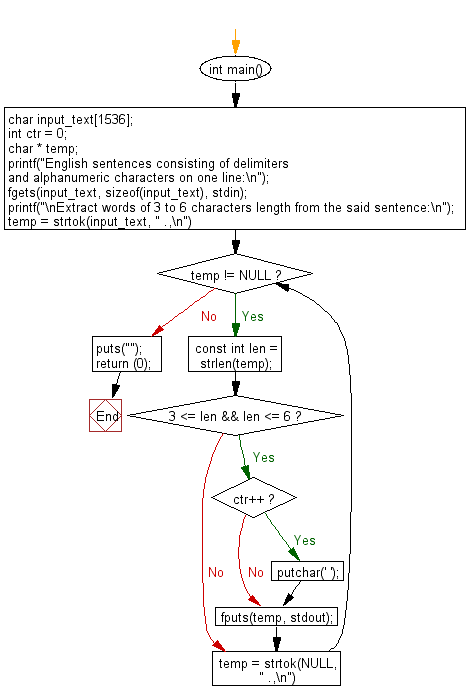
For more Practice: Solve these Related Problems:
- Write a C program to tokenize a sentence and print only words whose lengths are between 3 and 6 characters.
- Write a C program to use manual string parsing to extract and display words of a specific length range.
- Write a C program to iterate through a sentence and output words of 3 to 6 characters without using strtok.
- Write a C program to filter words by length from a given sentence and store the valid words in a separate array.
C programming Code Editor:
Previous: Write a C program to sum of all numerical values (positive integers) embedded in a sentence.
Next: Write a C program to find the number of combinations that satisfy p + q + r + s = n where n is a given number <= 4000 and p, q, r, s in the range of 0 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.