C Exercises: Find the number of combinations that satisfy p + q + r + s = n
Count combinations satisfying p+q+r+s=n
Write a C program to find the number of combinations that satisfy p + q + r + s = n where n is a given number <= 4000 and p, q, r, s are between 0 and 1000.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Define a macro to ensure non-negative numbers
#define check_num(x) (x>0?x:0)
// Define global variables
int n, result, x;
int main(void) {
// Prompt user for input
printf("Input a positive integer:\n");
// Read the input
scanf("%d", &n);
// Initialize result variable
result = 0;
// Loop through possible values of x within the specified range
for (x = check_num(n - 2000); x <= 2000 && n >= x; x++) {
// Calculate the result based on the given formula
result += (1001 - abs(1000 - (n - x))) * (1001 - abs(1000 - x));
}
// Print the number of combinations
printf("\nNumber of combinations of p,q,r,s:\n");
printf("%d\n", result);
// Return 0 to indicate successful execution
return 0;
}
Sample Output:
Input a positive integer: 25 Number of combinations of p,q,r,s: 3276
Flowchart:
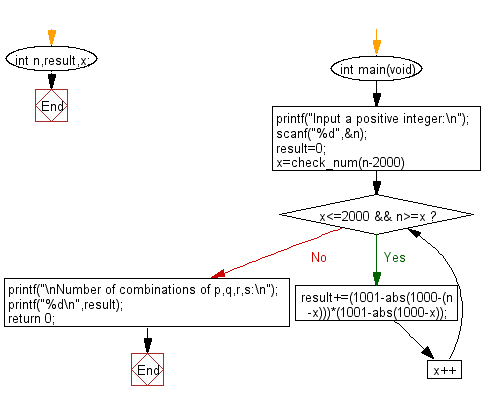
For more Practice: Solve these Related Problems:
- Write a C program to use four nested loops to count the number of quadruplets (p, q, r, s) that sum to n.
- Write a C program to implement a recursive solution that counts combinations of four numbers summing to n.
- Write a C program to use dynamic programming to count the number of ways four digits (0–1000) can sum to a given n.
- Write a C program to optimize the counting of quadruplet combinations with early loop termination when sums exceed n.
C programming Code Editor:
Previous: Write a C program to extract words of 3 to 6 characters length from a given sentence not more than 1024 characters.
Next: Write a C program, which adds up columns and rows of given table as shown in the following figure.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.