Check for valid triangle and calculate perimeter
Check for valid triangle and calculate perimeter
Write a C program that reads three floating-point values and checks if it is possible to make a triangle with them. Determine the perimeter of the triangle if the given values are valid.
Pictorial Presentation:
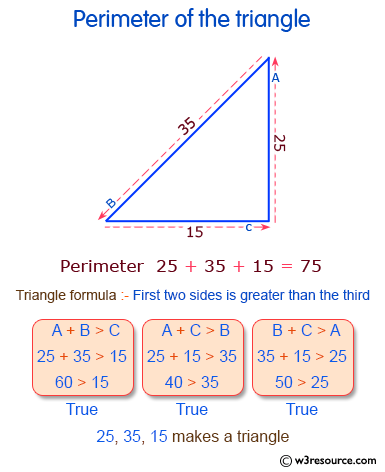
C Code:
#include <stdio.h>
int main() {
float x, y, z, P, A; // Declare variables for side lengths and perimeter
// Prompt user for the side lengths and store in 'x', 'y', and 'z'
printf("\nInput the first number: ");
scanf("%f", &x);
printf("\nInput the second number: ");
scanf("%f", &y);
printf("\nInput the third number: ");
scanf("%f", &z);
if(x < (y+z) && y < (x+z) && z < (y+x)) // Check if the sides can form a triangle
{
P = x+y+z; // Calculate perimeter
printf("\nPerimeter = %.1f\n", P); // Print perimeter
}
else
{
printf("Not possible to create a triangle..!"); // Print message if it's not possible to form a triangle
}
return 0;
}
Sample Output:
Input the first number: 25 Input the second number: 15 Input the third number: 35 Perimeter = 75.0
Flowchart:
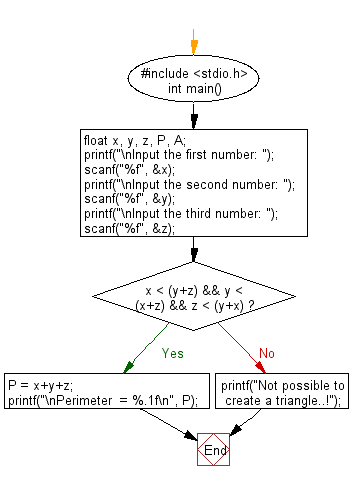
For more Practice: Solve these Related Problems:
- Write a C program to validate a triangle using side lengths and calculate its area using Heron’s formula if valid.
- Write a C program to check if three side lengths form a valid triangle and determine its type (scalene, isosceles, or equilateral).
- Write a C program to compute the perimeter of a triangle and its semi-perimeter for further geometric calculations.
- Write a C program to verify triangle validity using both side lengths and internal angles, then calculate the perimeter if valid.
C Programming Code Editor:
Previous: Write a C program that read 5 numbers and sum of all odd values between them.
Next: Write a C program that reads two integers and checks if they are multiplied or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.