Check if two integers are multiples
Check if two integers are multiples
Write a C program that reads two integers and checks whether they are multiplied or not.
Pictorial Presentation:
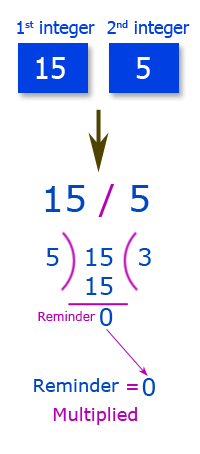
C Code:
#include <stdio.h>
int main() {
int x, y; // Declare variables for two integers
// Prompt user for the first number and store in 'x'
printf("\nInput the first number: ");
scanf("%d", &x);
// Prompt user for the second number and store in 'y'
printf("\nInput the second number: ");
scanf("%d", &y);
if(x > y)
{
int temp;
temp = x; // Swap the values of 'x' and 'y'
x = y;
y = temp;
}
if((y % x)== 0) // Check if 'x' is a factor of 'y'
{
printf("\nMultiplied!\n"); // Print message if 'x' is a factor of 'y'
}
else
{
printf("\nNot Multiplied!\n"); // Print message if 'x' is not a factor of 'y'
}
return 0;
}
Sample Output:
Input the first number: 5 Input the second number: 15 Multiplied!
Flowchart:
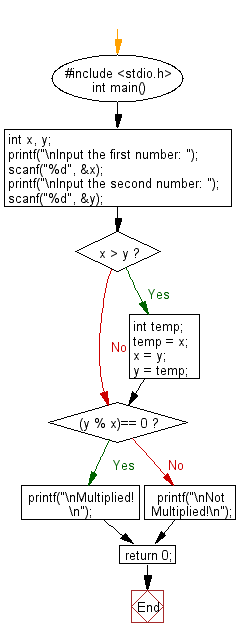
For more Practice: Solve these Related Problems:
- Write a C program to check if one integer is an exact divisor of another provided integer.
- Write a C program to determine if two integers are co-prime (i.e., have no common divisors other than 1).
- Write a C program to check if a given integer is a multiple of both 3 and 5 simultaneously.
- Write a C program to verify whether a pair of integers has any common multiple within a specified range.
C Programming Code Editor:
Previous: Write a C program that reads three floating values and check whether it is possible to make a triangle with them. Also calculate the perimeter of the triangle if the said values are valid.
Next: Write a C program that reads an integer between 1 and 12 and print the month of the year in English.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.