Print block 'F' and a large 'C'
Print block 'F' and a large 'C'
Write a C program to print a block F using the hash (#), where the F has a height of six characters and width of five and four characters. And also print a very large 'C'.
Block of 'F':
C Code:
#include <stdio.h>
int main()
{
// Print a line of hashes
printf("######\n");
// Print a single hash
printf("#\n");
// Print a single hash
printf("#\n");
// Print a line of hashes
printf("#####\n");
// Print a single hash
printf("#\n");
// Print a single hash
printf("#\n");
// Print a single hash
printf("#\n");
return(0);
}
Sample Output:
###### # # ##### # # #
Pictorial Presentation:
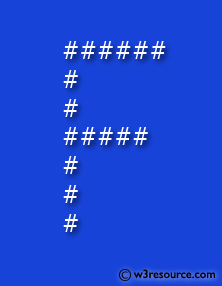
Flowchart:
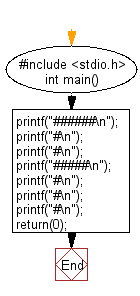
Print a big 'C':
C Code:
#include <stdio.h>
int main()
{
// Print top line of pattern
printf(" ######\n");
// Print second line of pattern
printf(" ## ##\n");
// Print lines 3 to 7 of pattern
printf(" #\n");
printf(" #\n");
printf(" #\n");
printf(" #\n");
printf(" #\n");
// Print bottom line of pattern
printf(" ## ##\n");
// Print last line of pattern
printf(" ######\n");
return(0);
}
Sample Output:
###### ## ## # # # # # ## ## ######
Pictorial Presentation:
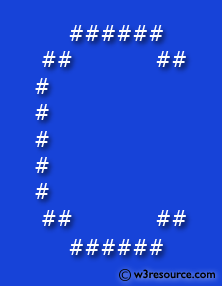
Flowchart:
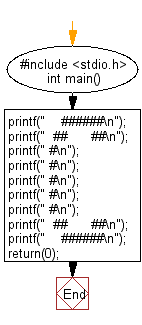
For more Practice: Solve these Related Problems:
- Write a C program to print a mirrored block letter 'F' using asterisks (*) with a hollow middle section.
- Write a C program to print a large, hollow 'C' with an inner space pattern and border highlights.
- Write a C program to print a rotated 'F' (90 degrees clockwise) with consistent block pattern style.
- Write a C program to print an alternating pattern combining block letters 'F' and 'C' in a zigzag arrangement.
C Programming Code Editor:
Previous: Write a C program to get the C version you are using.
Next: Write a C program to print the following characters in a reverse way.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.