Print block 'F' and a large 'C'
Reverse characters ('X', 'M', 'L')
Write a C program to print the following characters in reverse.
Pictorial Presentation:
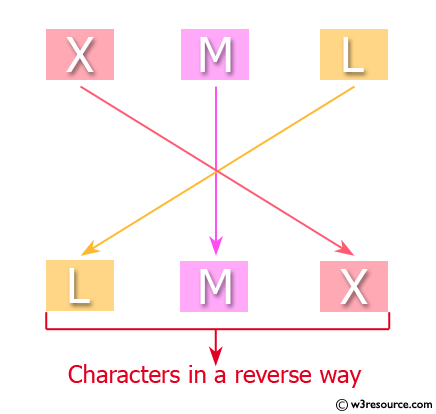
Test Characters : 'X', 'M', 'L'
C Code:
#include <stdio.h>
int main()
{
// Declare and initialize character variables
char char1 = 'X';
char char2 = 'M';
char char3 = 'L';
// Print the original and reversed characters
printf("The reverse of %c%c%c is %c%c%c\n",
char1, char2, char3,
char3, char2, char1);
return(0);
}
Explanation:
In the exercise above -
#include <stdio.h> - This code includes the standard input/output library <stdio.h>.
- In the "main()" function, it declares three character variables: 'char1', 'char2', and 'char3', and assigns them the values 'X', 'M', and 'L' respectively.
- It uses the "printf()" function to display a formatted message. The message contains placeholders specified by %c, which represent characters.
- Inside the "printf()" function, it provides the values to substitute for the placeholders. In this case, it provides 'char1', 'char2', and 'char3' followed by their reverse order: 'char3', 'char2', and 'char1'.
- The program will print: "The reverse of XML is LMX" because it swaps characters in reverse order.
- Finally, the main function returns 0 to indicate successful program execution.
Sample Output:
The reverse of XML is LMX
Flowchart:
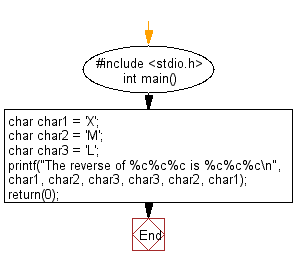
For more Practice: Solve these Related Problems:
- Write a C program to reverse a string entered by the user without using any library functions.
- Write a C program to reverse the order of words in a sentence while preserving the word content.
- Write a C program to reverse only the vowels in a string while keeping other characters in place.
- Write a C program to recursively reverse a given string and print the result.
C Programming Code Editor:
Previous: Write a C program to print a big 'C'.
Next: Write a C program to compute the perimeter and area of a rectangle with a height of 7 inches. and width of 5 inches.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.